<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication1;assembly="
Title="WPF" Height="248" Width="128">
<Window.Resources>
<local:Countries x:Key="countries"/>
<Style x:Key="AlternateStyle">
<Setter Property="ListBoxItem.Background" Value="LightGray"/>
</Style>
</Window.Resources>
<Grid>
<ListBox DisplayMemberPath="Name" ItemsSource="{Binding Source={StaticResource countries}}" >
<ListBox.ItemContainerStyleSelector>
<local:AlternatingRowStyleSelector AlternateStyle="{StaticResource AlternateStyle}" />
</ListBox.ItemContainerStyleSelector>
</ListBox>
</Grid>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Controls;
using System.Collections.ObjectModel;
namespace WpfApplication1
{
public class AlternatingRowStyleSelector : StyleSelector
{
public Style DefaultStyle
{
get;
set;
}
public Style AlternateStyle
{
get;
set;
}
private bool isAlternate = false;
public override Style SelectStyle(object item, DependencyObject container)
{
Style style = isAlternate ? AlternateStyle : DefaultStyle;
isAlternate = !isAlternate;
return style;
}
}
public class Country
{
private string name;
private Continent continent;
public Country(string name, Continent continent)
{
this.name = name;
this.continent = continent;
}
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
public Continent Continent
{
get
{
return continent;
}
set
{
continent = value;
}
}
}
public enum Continent
{
Europe,
NorthAmerica,
}
public class Countries : Collection<Country>
{
public Countries()
{
this.Add(new Country("Great Britan", Continent.Europe));
this.Add(new Country("USA", Continent.NorthAmerica));
this.Add(new Country("Canada", Continent.NorthAmerica));
}
}
}
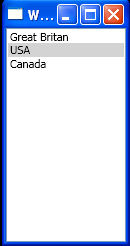