<Window x:Class="ColorConverter.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:ColorConverter"
Title="Colors" Height="400" Width="400">
<Window.Resources>
<local:ColorMultiConverter x:Key="colorConverter"/>
<local:ColorNameValueConverter x:Key="colorNameConverter"/>
</Window.Resources>
<Grid>
<Label Name="label1">Red:</Label>
<Slider Name="redSlider"/>
<Label Name="label2">Green:</Label>
<Slider Name="greenSlider"/>
<Label Name="label3">Blue:</Label>
<Slider Name="blueSlider"/>
<Grid Name="colorBlock">
<Grid.Background>
<SolidColorBrush>
<SolidColorBrush.Color>
<MultiBinding Converter="{StaticResource colorConverter}">
<Binding ElementName="redSlider" Path="Value"/>
<Binding ElementName="greenSlider" Path="Value"/>
<Binding ElementName="blueSlider" Path="Value"/>
</MultiBinding>
</SolidColorBrush.Color>
</SolidColorBrush>
</Grid.Background>
<Label HorizontalContentAlignment="Center" VerticalContentAlignment="Center" VerticalAlignment="Center">
<Label.Foreground>
<SolidColorBrush>
<SolidColorBrush.Color>
<MultiBinding Converter="{StaticResource colorConverter}" ConverterParameter="inverse">
<Binding ElementName="redSlider" Path="Value"/>
<Binding ElementName="greenSlider" Path="Value"/>
<Binding ElementName="blueSlider" Path="Value"/>
</MultiBinding>
</SolidColorBrush.Color>
</SolidColorBrush>
</Label.Foreground>
<TextBlock>
<TextBlock.Text>
<MultiBinding StringFormat="Red={0}, Green={1}, Blue={2}">
<Binding ElementName="redSlider" Path="Value" />
<Binding ElementName="greenSlider" Path="Value" />
<Binding ElementName="blueSlider" Path="Value" />
</MultiBinding>
</TextBlock.Text>
</TextBlock>
</Label>
</Grid>
<TextBox>
<TextBox.Text>
<MultiBinding StringFormat=" R{0} G{1} B{2}">
<Binding ElementName="redSlider" Path="Value" />
<Binding ElementName="greenSlider" Path="Value" />
<Binding ElementName="blueSlider" Path="Value" />
</MultiBinding>
</TextBox.Text>
</TextBox>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Collections.Generic;
using System.Reflection;
namespace ColorConverter
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void colorBlock_MouseDown(object sender, MouseButtonEventArgs e)
{
Random rnd = new Random((int)DateTime.Now.Ticks);
Color newColor = Color.FromRgb((byte)rnd.Next(255), (byte)rnd.Next(255), (byte)rnd.Next(255));
colorBlock.Background = new SolidColorBrush(newColor);
}
}
class ColorMultiConverter : IMultiValueConverter
{
public object Convert(object[] values, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
bool inverse = (parameter != null) && (string.Compare(parameter.ToString(), "inverse", true) == 0);
byte R = System.Convert.ToByte((double)values[0]);
byte G = System.Convert.ToByte((double)values[1]);
byte B = System.Convert.ToByte((double)values[2]);
Color newColor;
if (inverse)
newColor = Color.FromRgb((byte)(255 - R), (byte)(255 - G), (byte)(255 - B));
else
newColor = Color.FromRgb(R, G, B);
return newColor;
}
public object[] ConvertBack(object value, Type[] targetTypes, object parameter, System.Globalization.CultureInfo culture)
{
Color oldColor = (Color)value;
double R = (double)oldColor.R;
double G = (double)oldColor.G;
double B = (double)oldColor.B;
return new object[] { R, G, B };
}
}
class ColorNameValueConverter : IValueConverter
{
private Dictionary<Color, string> namedColors = new Dictionary<Color, string>();
public ColorNameValueConverter()
{
PropertyInfo[] colorProperties = typeof(Colors).GetProperties(BindingFlags.Static | BindingFlags.Public);
Color stepColor;
foreach (PropertyInfo pi in colorProperties)
{
if (pi.PropertyType == typeof(Color))
{
stepColor = (Color)pi.GetValue(null, null);
namedColors[stepColor] = pi.Name;
}
}
}
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
Color col = (Color)value;
if(namedColors.ContainsKey(col))
return namedColors[col];
return DependencyProperty.UnsetValue;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
throw new NotImplementedException();
}
}
}
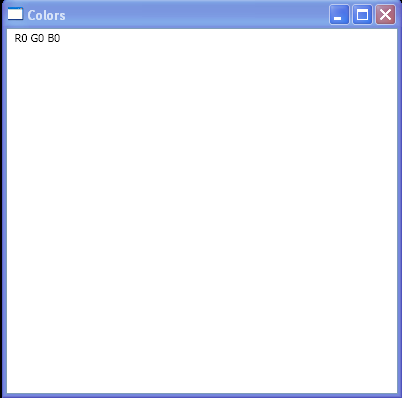