<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
x:Class="WpfApplication1.Window1"
Title="ColumnDefinitions Sample">
<Border BorderBrush="Black" Background="White" BorderThickness="2">
<DockPanel Margin="10,0,0,0">
<TextBlock FontSize="20" FontWeight="Bold" DockPanel.Dock="Top">Grid Column and Row Collections</TextBlock>
<Grid DockPanel.Dock="Top" HorizontalAlignment="Left" Name="grid1" ShowGridLines="true" Width="625" Height="400">
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition/>
<RowDefinition/>
<RowDefinition/>
</Grid.RowDefinitions>
</Grid>
<StackPanel HorizontalAlignment="Left" Orientation="Horizontal" Width="625" DockPanel.Dock="Top">
<Button Width="125" Click="containsCol">Contains Column?</Button>
</StackPanel>
</DockPanel>
</Border>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
namespace WpfApplication1
{
public partial class Window1 : Window
{
RowDefinition rowDef1;
ColumnDefinition colDef1;
private void containsCol(object sender, RoutedEventArgs e)
{
colDef1 = new ColumnDefinition();
grid1.ColumnDefinitions.Insert(grid1.ColumnDefinitions.Count, colDef1);
Console.WriteLine(grid1.ColumnDefinitions.IndexOf(colDef1).ToString());
if (grid1.ColumnDefinitions.Contains(colDef1))
{
Console.WriteLine("Grid Contains ColumnDefinition colDef1");
}
else
{
Console.WriteLine("Grid Does Not Contain ColumnDefinition colDef1");
}
}
}
}
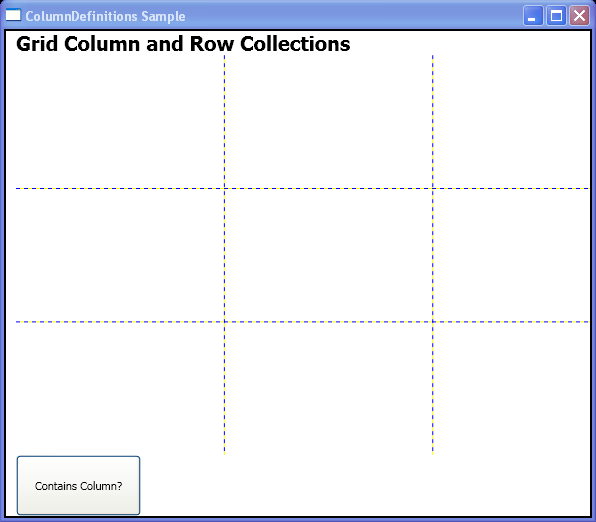