<Window x:Class="WpfApplication1.StoryboardInCode"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Storyboard Animation in Code" Height="300" Width="300">
<Canvas>
<Button Content="Button1" Width="150" Height="80"
Canvas.Left="50" Canvas.Top="20">
<Button.Background>
<SolidColorBrush x:Name="brush1" />
</Button.Background>
</Button>
<Button Content="Button2" Width="150" Height="80"
Canvas.Left="50" Canvas.Top="110">
<Button.Background>
<SolidColorBrush x:Name="brush2" />
</Button.Background>
</Button>
</Canvas>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Shapes;
using System.Windows.Media.Animation;
namespace WpfApplication1
{
public partial class StoryboardInCode : Window
{
public StoryboardInCode()
{
InitializeComponent();
Storyboard sb = new Storyboard();
ColorAnimation ca1 = new ColorAnimation(Colors.Blue, Colors.Yellow,new Duration(new TimeSpan(0, 0, 10)));
ca1.RepeatBehavior = RepeatBehavior.Forever;
ca1.AutoReverse = true;
Storyboard.SetTargetName(ca1, "brush1");
Storyboard.SetTargetProperty(ca1,new PropertyPath(SolidColorBrush.ColorProperty));
ColorAnimation ca2 = new ColorAnimation(Colors.Red, Colors.Green,new Duration(new TimeSpan(0, 0, 10)));
ca2.RepeatBehavior = RepeatBehavior.Forever;
ca2.AutoReverse = true;
ca2.BeginTime = new TimeSpan(0, 0, 5);
Storyboard.SetTargetName(ca2, "brush2");
Storyboard.SetTargetProperty(ca2,new PropertyPath(SolidColorBrush.ColorProperty));
sb.Children.Add(ca1);
sb.Children.Add(ca2);
sb.Begin(this);
}
}
}
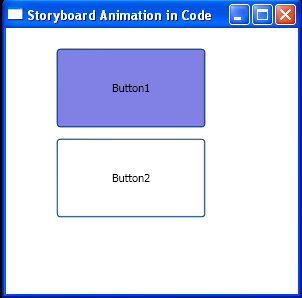