<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication1"
xmlns:compModel="clr-namespace:System.ComponentModel;assembly=WindowsBase"
xmlns:data="clr-namespace:System.Windows.Data;assembly=PresentationFramework"
Title="CollectionViewSourceBinding" Height="300" Width="300">
<Window.Resources>
<local:People x:Key="Family">
<local:Employee Name="A" Age="11" />
<local:Employee Name="B" Age="12" />
<local:Employee Name="C" Age="28" />
<local:Employee Name="D" Age="88" />
</local:People>
<local:AgeToRangeConverter x:Key="ageConverter" />
<CollectionViewSource x:Key="SortedGroupedFamily" Source="{StaticResource Family}">
<CollectionViewSource.SortDescriptions>
<compModel:SortDescription PropertyName="Name" Direction="Ascending" />
<compModel:SortDescription PropertyName="Age" Direction="Descending" />
</CollectionViewSource.SortDescriptions>
<CollectionViewSource.GroupDescriptions>
<data:PropertyGroupDescription PropertyName="Age" Converter="{StaticResource ageConverter}" />
<data:PropertyGroupDescription PropertyName="Age" />
</CollectionViewSource.GroupDescriptions>
</CollectionViewSource>
</Window.Resources>
<Grid>
<ListBox ItemsSource="{Binding Source={StaticResource SortedGroupedFamily}}" DisplayMemberPath="Name">
<ListBox.GroupStyle>
<x:Static Member="GroupStyle.Default" />
</ListBox.GroupStyle>
</ListBox>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.ComponentModel;
using System.Collections.ObjectModel;
namespace WpfApplication1 {
public class Employee : INotifyPropertyChanged {
public event PropertyChangedEventHandler PropertyChanged;
protected void Notify(string propName) {
if( this.PropertyChanged != null ) {
PropertyChanged(this, new PropertyChangedEventArgs(propName));
}
}
string name;
public string Name {
get { return this.name; }
set {
if( this.name == value ) { return; }
this.name = value;
Notify("Name");
}
}
int age;
public int Age {
get { return this.age; }
set {
if( this.age == value ) { return; }
this.age = value;
Notify("Age");
}
}
public Employee() { }
public Employee(string name, int age) {
this.name = name;
this.age = age;
}
}
class People : ObservableCollection<Employee> { }
public partial class Window1 : System.Windows.Window {
public Window1() {
InitializeComponent();
}
}
public class AgeToRangeConverter : IValueConverter {
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
return (int)value < 25 ? "Under 25" : "Over 25";
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
throw new NotImplementedException();
}
}
}
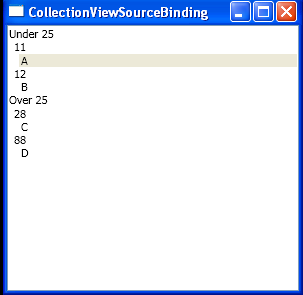