<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" Height="100" Width="300">
<StackPanel>
<ComboBox Name="comboBox" IsEditable="True" Margin="5" SelectionChanged="ComboBox_SelectionChanged">
<ComboBoxItem Content="ComboBox Item 1" Selected="ComboBoxItem_Selected" />
<ComboBoxItem Content="ComboBox Item 2" Selected="ComboBoxItem_Selected" />
<ComboBoxItem Content="ComboBox Item 3" Selected="ComboBoxItem_Selected" IsSelected="True"/>
<ComboBoxItem Content="ComboBox Item 4" Selected="ComboBoxItem_Selected" />
<ComboBoxItem Content="ComboBox Item 5" Selected="ComboBoxItem_Selected" />
</ComboBox>
<Button Content="Get Selected" Margin="5" Width="100" Click="Button_Click" />
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
ComboBoxItem item = comboBox.SelectedItem as ComboBoxItem;
if (item != null)
{
MessageBox.Show("Current item: " + item.Content, Title);
}
else if (!String.IsNullOrEmpty(comboBox.Text))
{
MessageBox.Show("Text entered: " + comboBox.Text, Title);
}
}
private void ComboBox_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
if (!IsInitialized) return;
ComboBoxItem item = comboBox.SelectedItem as ComboBoxItem;
if (item != null)
{
MessageBox.Show("Selected item: " + item.Content, Title);
}
}
private void ComboBoxItem_Selected(object sender,RoutedEventArgs e)
{
if (!IsInitialized) return;
ComboBoxItem item = e.OriginalSource as ComboBoxItem;
if (item != null)
{
MessageBox.Show(item.Content + " was selected.", Title);
}
}
}
}
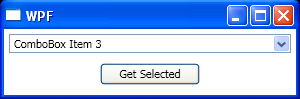