<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication1"
Title="XmlBinding" Height="325" Width="400">
<Window.Resources>
<local:AgeToForegroundConverter x:Key="ageConverter" />
</Window.Resources>
<StackPanel Name="stackPanel">
<ListBox ItemsSource="{Binding}" IsSynchronizedWithCurrentItem="True">
<ListBox.GroupStyle>
<x:Static Member="GroupStyle.Default" />
</ListBox.GroupStyle>
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock>
<TextBlock Text="{Binding XPath=@Name}" />
(age: <TextBlock Text="{Binding XPath=@Age}" Foreground="{Binding XPath=@Age, Converter={StaticResource ageConverter}}" />)
</TextBlock>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
<TextBlock>Name:</TextBlock>
<TextBox Text="{Binding XPath=@Name}" />
<TextBlock>Age:</TextBlock>
<TextBox Text="{Binding XPath=@Age}" Foreground="{Binding XPath=@Age, Converter={StaticResource ageConverter}}" />
<Button Name="birthdayButton">Birthday</Button>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.Collections.Generic;
using System.Diagnostics;
using System.Collections;
using System.Data;
using System.Data.OleDb;
using System.ComponentModel;
using System.Xml;
namespace WpfApplication1
{
public class AgeToForegroundConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
Debug.Assert(targetType == typeof(Brush));
int age = int.Parse(value.ToString());
return (age > 60 ? Brushes.Red : Brushes.Black);
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
throw new NotImplementedException();
}
}
public partial class Window1 : Window
{
XmlDocument doc;
public Window1()
{
InitializeComponent();
doc = new XmlDocument();
doc.LoadXml(@"<Company xmlns='http://company.com'>
<Employee Name='A' Age='61' />
<Employee Name='B' Age='72' />
<Employee Name='C' Age='38' />
</Company>");
XmlNamespaceManager manager = new XmlNamespaceManager(doc.NameTable);
manager.AddNamespace("sb", "http://company.com");
Binding.SetXmlNamespaceManager(stackPanel, manager);
Binding b = new Binding();
b.XPath = "/sb:Company/sb:Employee";
b.Source = doc;
stackPanel.SetBinding(StackPanel.DataContextProperty, b);
this.birthdayButton.Click += birthdayButton_Click;
}
void birthdayButton_Click(object sender, RoutedEventArgs e) {
ICollectionView view = CollectionViewSource.GetDefaultView(stackPanel.DataContext);
XmlElement person = (XmlElement)view.CurrentItem;
person.SetAttribute("Age",(int.Parse(person.Attributes["Age"].Value) + 1).ToString());
Console.WriteLine(person.Attributes["Name"].Value);
Console.WriteLine(person.Attributes["Age"].Value);
}
}
}
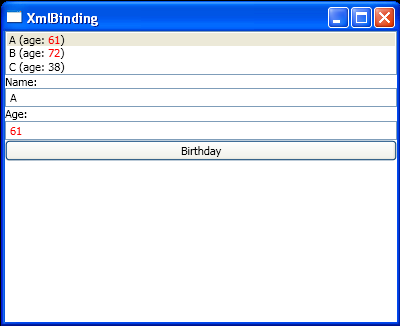