<Window x:Class="GridSplitter_Example.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="GridSplitter Example">
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Name="Col0" />
<ColumnDefinition Name="Col1" />
<ColumnDefinition Name="Col2" />
<ColumnDefinition Name="Col3" />
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
<RowDefinition Name="Row0" />
<RowDefinition Name="Row1" />
<RowDefinition Name="Row2" />
</Grid.RowDefinitions>
<StackPanel Grid.Row="0" Grid.Column="0" Background="Orange">
<TextBlock>Row 0 Col 0</TextBlock>
</StackPanel>
<StackPanel Grid.Row="1" Grid.Column="0" Background="Blue">
<TextBlock>Row 1 Col 0</TextBlock>
</StackPanel>
<StackPanel Grid.Row="2" Grid.Column="0" Background="Green">
<TextBlock>Row 2 Col 1</TextBlock>
</StackPanel>
<StackPanel Grid.Row="0" Grid.Column="1" Background="Purple">
<TextBlock>Row 0 Col 1</TextBlock>
</StackPanel>
<StackPanel Grid.Row="1" Grid.Column="1" Background="Red">
<TextBlock>Row 1 Col 1</TextBlock>
</StackPanel>
<StackPanel Grid.Row="2" Grid.Column="1" Background="Salmon">
<TextBlock>Row 2 Col 1</TextBlock>
</StackPanel>
<StackPanel Grid.Row="0" Grid.Column="2" Background="MediumVioletRed">
<TextBlock>Row 0 Col 2</TextBlock>
</StackPanel>
<StackPanel Grid.Row="1" Grid.Column="2" Background="SteelBlue">
<TextBlock>Row 1 Col 2</TextBlock>
</StackPanel>
<StackPanel Grid.Row="2" Grid.Column="2" Background="Olive">
<TextBlock>Row 2 Col 2</TextBlock>
</StackPanel>
<GridSplitter Name="myGridSplitter" Grid.Column="1" Grid.Row="1" Width="5"/>
<StackPanel Grid.Column="3" Grid.RowSpan="3" Background="Brown">
<TextBlock FontSize="14" Foreground="Yellow">Property Settings</TextBlock>
<StackPanel Margin="0,5,0,0">
<TextBlock>GridResizeBehavior</TextBlock>
<RadioButton Name="BehaviorBasedOnAlignment" Checked="ResizeBehaviorChanged" IsChecked="true" GroupName="GridResizeBehaviorProperty">
BasedOnAlignment (default)
</RadioButton>
<RadioButton Name="BehaviorCurrentAndNext" Checked="ResizeBehaviorChanged" GroupName="GridResizeBehaviorProperty">
CurrentAndNext
</RadioButton>
<RadioButton Name="BehaviorPreviousAndCurrent" Checked="ResizeBehaviorChanged" GroupName="GridResizeBehaviorProperty">
PreviousAndCurrent
</RadioButton>
<RadioButton Name="BehaviorPreviousAndNext" Checked="ResizeBehaviorChanged" GroupName="GridResizeBehaviorProperty">
PreviousAndNext
</RadioButton>
</StackPanel>
</StackPanel>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Media;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace GridSplitter_Example
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void ResizeBehaviorChanged(object sender, RoutedEventArgs e)
{
if ((Boolean)BehaviorBasedOnAlignment.IsChecked)
myGridSplitter.ResizeBehavior = GridResizeBehavior.BasedOnAlignment;
else if ((Boolean)BehaviorCurrentAndNext.IsChecked)
myGridSplitter.ResizeBehavior = GridResizeBehavior.CurrentAndNext;
else if ((Boolean)BehaviorPreviousAndCurrent.IsChecked)
myGridSplitter.ResizeBehavior = GridResizeBehavior.PreviousAndCurrent;
else if ((Boolean)BehaviorPreviousAndNext.IsChecked)
myGridSplitter.ResizeBehavior = GridResizeBehavior.PreviousAndNext;
}
}
}
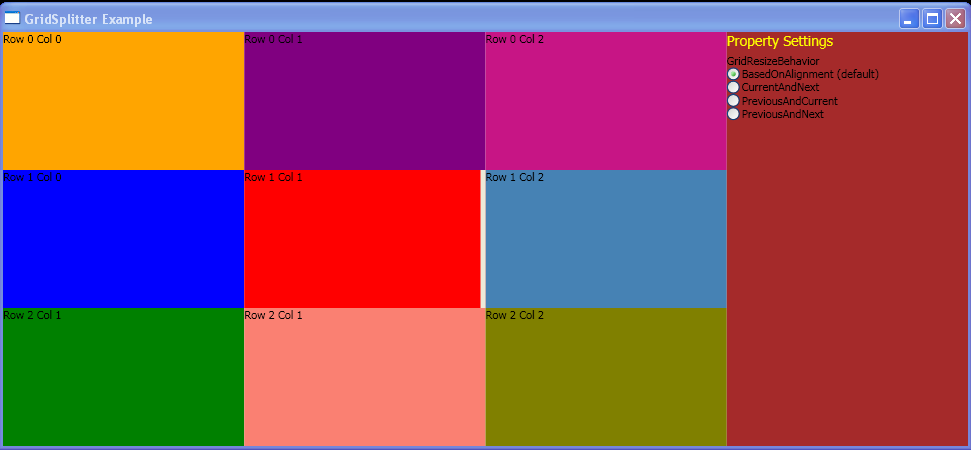