<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:sys="clr-namespace:System;assembly=mscorlib"
xmlns:local="clr-namespace:WpfApplication1"
Title="Random Numbers Built to Order">
<Window.Resources>
<ObjectDataProvider x:Key="nextRandomNumber" ObjectType="{x:Type sys:Random}" MethodName="Next">
<ObjectDataProvider.MethodParameters>
<sys:Int32>0</sys:Int32>
<sys:Int32>10</sys:Int32>
</ObjectDataProvider.MethodParameters>
</ObjectDataProvider>
<local:StringToInt32Converter x:Key="numConverter" />
</Window.Resources>
<StackPanel DataContext="{StaticResource nextRandomNumber}">
<Label>Min</Label>
<TextBox Name="minTextBox" ToolTip="{Binding ElementName=minTextBox, Path=(Validation.Errors)[0].ErrorContent}">
<TextBox.Text>
<Binding Path="MethodParameters[0]" BindsDirectlyToSource="True" Converter="{StaticResource numConverter}">
<Binding.ValidationRules>
<local:IntegerValidator />
</Binding.ValidationRules>
</Binding>
</TextBox.Text>
</TextBox>
<Label>Max</Label>
<TextBox Name="maxTextBox" ToolTip="{Binding ElementName=maxTextBox, Path=(Validation.Errors)[0].ErrorContent}">
<TextBox.Text>
<Binding Path="MethodParameters[1]" BindsDirectlyToSource="True" Converter="{StaticResource numConverter}">
<Binding.ValidationRules>
<local:IntegerValidator />
</Binding.ValidationRules>
</Binding>
</TextBox.Text>
</TextBox>
<Label>Random</Label>
<TextBox Text="{Binding Mode=OneTime}" IsReadOnly="True" />
<Button Name="nextButton">Next</Button>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace WpfApplication1 {
public partial class Window1 : System.Windows.Window {
public Window1() {
InitializeComponent();
nextButton.Click += new RoutedEventHandler(nextButton_Click);
}
void nextButton_Click(object sender, RoutedEventArgs e) {
((ObjectDataProvider)Resources["nextRandomNumber"]).Refresh();
}
}
public class StringToInt32Converter : IValueConverter {
public object Convert(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
return ((int)value).ToString();
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture) {
return int.Parse((string)value);
}
}
public class IntegerValidator : ValidationRule {
public override ValidationResult Validate(object value, System.Globalization.CultureInfo cultureInfo) {
try {
int.Parse((string)value);
return ValidationResult.ValidResult;
}
catch( Exception ex ) {
return new ValidationResult(false, ex.Message);
}
}
}
}
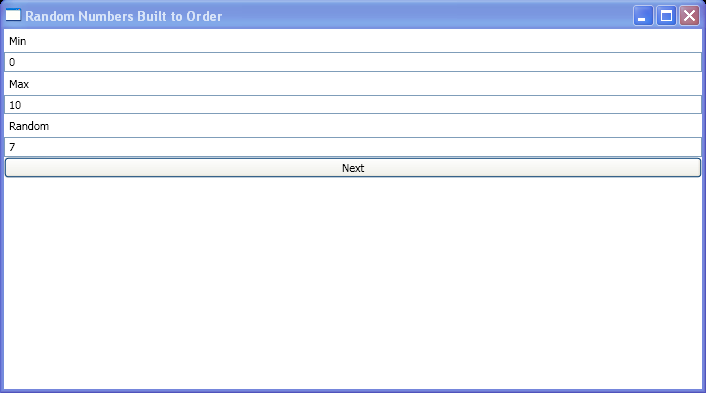