<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApplication1"
Title="ManualUpdateTarget" Height="135" Width="200">
<Window.Resources>
<local:Person x:Key="Tom" Name="Tom" Age="11" />
</Window.Resources>
<StackPanel DataContext="{StaticResource Tom}">
<TextBlock Margin="5" VerticalAlignment="Center">Name:</TextBlock>
<TextBox Margin="5" Name="nameTextBox" Text="{Binding Path=Name}" />
<TextBlock Margin="5" VerticalAlignment="Center">Age:</TextBlock>
<TextBox Margin="5" Name="ageTextBox" Text="{Binding Path=Age}" />
<Button Margin="5" Width="75" Name="birthdayButton">Birthday</Button>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.ComponentModel;
namespace WpfApplication1 {
public class Person {
string name;
public string Name {
get { return this.name; }
set {
if( this.name == value ) { return; }
this.name = value;
}
}
int age;
public int Age {
get { return this.age; }
set {
if( this.age == value ) { return; }
this.age = value;
}
}
public Person() { }
public Person(string name, int age) {
this.name = name;
this.age = age;
}
}
public partial class Window1 : System.Windows.Window {
public Window1() {
InitializeComponent();
this.birthdayButton.Click += birthdayButton_Click;
}
void birthdayButton_Click(object sender, RoutedEventArgs e) {
Person person = (Person)this.FindResource("Tom");
person.Age = person.Age+1;
BindingOperations.GetBindingExpression(ageTextBox, TextBox.TextProperty).UpdateTarget();
Console.WriteLine(person.Name);
Console.WriteLine(person.Age);
}
}
}
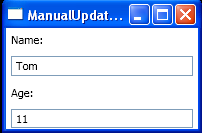