<Window x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF" SizeToContent="Height" Width="300">
<Grid Name="grid">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition/>
<ColumnDefinition/>
</Grid.ColumnDefinitions>
<Border Grid.Column="0" BorderBrush="Gray" BorderThickness="1" />
<Border Grid.Column="1" BorderBrush="Gray" BorderThickness="1" />
<StackPanel Grid.Column="0" HorizontalAlignment="Center" Margin="5" Name="spLeftContainer">
<TextBlock FontSize="16" Text="Radio Group 1" />
<RadioButton Content="Radio Button 1A" GroupName="Group1" IsChecked="True" Margin="5" Name="rbnOneA" />
<RadioButton Content="Radio Button 1B" GroupName="Group1" Margin="5" Name="rbnOneB" />
<RadioButton Content="Radio Button 1C" GroupName="Group1" Margin="5" Name="rbnOneC" />
<Separator/>
<TextBlock FontSize="16" Text="Radio Group 2" />
<RadioButton Checked="RadioButton_Checked" GroupName="Group2" Content="Radio Button 2A" IsChecked="True"
Margin="5" Name="rbnTwoA" />
<RadioButton Checked="RadioButton_Checked" GroupName="Group2" Content="Radio Button 2B" Margin="5" Name="rbnTwoB"/>
<RadioButton Checked="RadioButton_Checked" GroupName="Group2" Content="Radio Button 2C" Margin="5" Name="rbnTwoC"/>
</StackPanel>
<StackPanel Grid.Column="1" HorizontalAlignment="Center" Margin="5" Name="spRightContainer">
<TextBlock FontSize="16" Text="Radio Group 1" />
<RadioButton Content="Radio Button 1D" GroupName="Group1" Margin="5" Name="rbnOneD" />
<RadioButton Content="Radio Button 1E" GroupName="Group1" Margin="5" Name="rbnOneE" />
</StackPanel>
<Button Content="Show Group1 Selection" Grid.ColumnSpan="2" Grid.Row="1" HorizontalAlignment="Center"
Margin="10" MaxHeight="25" Click="Button_Click" />
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Linq;
using System.Windows;
using System.Windows.Controls;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
RadioButton radioButton = null;
radioButton = GetCheckedRadioButton(spLeftContainer.Children, "Group1");
if (radioButton == null)
{
radioButton = GetCheckedRadioButton(spRightContainer.Children, "Group1");
}
MessageBox.Show(radioButton.Content + " checked.", Title);
}
private RadioButton GetCheckedRadioButton(UIElementCollection children, String groupName)
{
return children.OfType<RadioButton>().FirstOrDefault( rb => rb.IsChecked == true && rb.GroupName == groupName);
}
private void RadioButton_Checked(object sender, RoutedEventArgs e)
{
if (!this.IsInitialized) return;
RadioButton radioButton = e.OriginalSource as RadioButton;
if (radioButton != null)
{
MessageBox.Show(radioButton.Content + " checked.", Title);
}
}
}
}
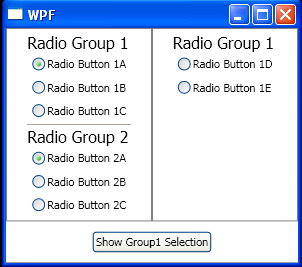