<Window
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:c="clr-namespace:WpfApplication1"
x:Class="WpfApplication1.Window1"
Title="Binding Validation Sample"
SizeToContent="WidthAndHeight"
ResizeMode="NoResize">
<Window.Resources>
<c:MyDataSource x:Key="ods"/>
</Window.Resources>
<StackPanel>
<TextBox Name="textBox2" Width="50" FontSize="15">
<TextBox.Text>
<Binding Path="Age2" Source="{StaticResource ods}" UpdateSourceTrigger="PropertyChanged" >
<Binding.ValidationRules>
<c:AgeRangeRule Min="21" Max="30"/>
</Binding.ValidationRules>
</Binding>
</TextBox.Text>
</TextBox>
</StackPanel>
</Window>
//File:Window.xaml.cs
using System;
using System.ComponentModel;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Navigation;
using System.Windows.Shapes;
using System.Windows.Data;
using System.Globalization;
using System.Collections.ObjectModel;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
}
public class AgeRangeRule : ValidationRule
{
private int _min;
private int _max;
public AgeRangeRule()
{
}
public int Min
{
get { return _min; }
set { _min = value; }
}
public int Max
{
get { return _max; }
set { _max = value; }
}
public override ValidationResult Validate(object value, CultureInfo cultureInfo)
{
int age = 0;
try
{
if (((string)value).Length > 0)
age = Int32.Parse((String)value);
}
catch (Exception e)
{
return new ValidationResult(false, "Illegal characters or " + e.Message);
}
if ((age < Min) || (age > Max))
{
return new ValidationResult(false,
"Please enter an age in the range: " + Min + " - " + Max + ".");
}
else
{
return new ValidationResult(true, null);
}
}
}
public class MyDataSource
{
private int _age;
private int _age2;
private int _age3;
public MyDataSource()
{
Age = 0;
Age2 = 0;
}
public int Age
{
get { return _age; }
set { _age = value; }
}
public int Age2
{
get { return _age2; }
set { _age2 = value; }
}
public int Age3
{
get { return _age3; }
set { _age3 = value; }
}
}
}
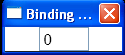