<Window
x:Class="WpfApplication1.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="600" Width="800">
<DockPanel>
<StackPanel DockPanel.Dock="Bottom" Orientation="Horizontal">
<TextBox x:Name="tbxInsertionText" Width="200" Margin="5,0" />
<Button DockPanel.Dock="Bottom" Content="Insert" Click="btnInsert_Click"/>
</StackPanel>
<RichTextBox x:Name="rtbTextContent" />
</DockPanel>
</Window>
//File:Window.xaml.cs
using System.Windows;
using System.Windows.Documents;
using System.Windows.Input;
namespace WpfApplication1
{
public partial class Window1 : Window
{
public Window1()
{
InitializeComponent();
}
private void btnInsert_Click(object sender, RoutedEventArgs e)
{
if (string.IsNullOrEmpty(tbxInsertionText.Text))
{
return;
}
rtbTextContent.BeginChange();
if (rtbTextContent.Selection.Text != string.Empty)
{
rtbTextContent.Selection.Text = string.Empty;
}
TextPointer tp = rtbTextContent.CaretPosition.GetPositionAtOffset(0, LogicalDirection.Forward);
rtbTextContent.CaretPosition.InsertTextInRun(tbxInsertionText.Text);
rtbTextContent.CaretPosition = tp;
rtbTextContent.EndChange();
Keyboard.Focus(rtbTextContent);
}
}
}
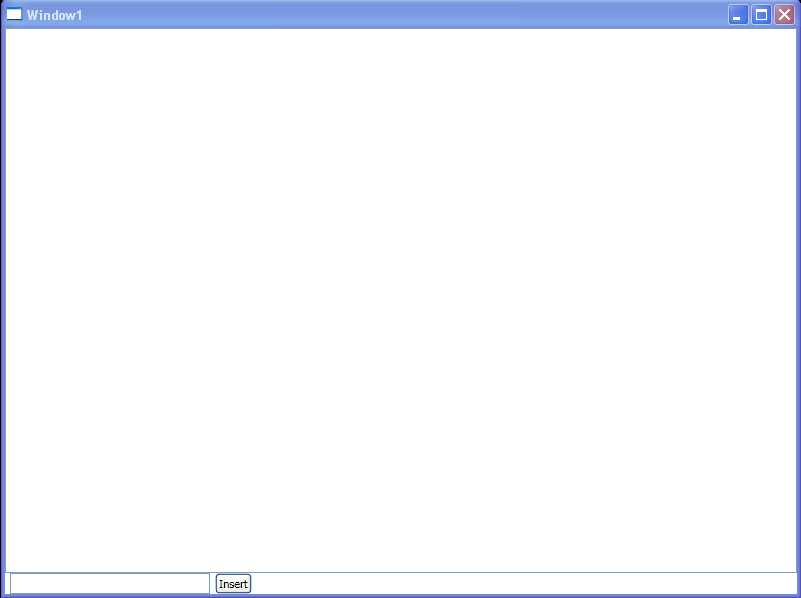