<Window x:Class="BackgroundWorkerExample.MyWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="BackgroundWorkerExample" Height="300" Width="300">
<Grid>
</Grid>
</Window>
//File:Window.xaml.cs
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
using System.ComponentModel;
using System.Threading;
namespace BackgroundWorkerExample
{
partial class MyWindow : Window
{
BackgroundWorker bw = new BackgroundWorker();
public MyWindow()
{
bw.DoWork += new DoWorkEventHandler(bw_DoWork);
bw.ProgressChanged += bw_ProgressChanged;
bw.RunWorkerCompleted += bw_RunWorkerCompleted;
bw.WorkerReportsProgress = true;
bw.RunWorkerAsync();
}
void bw_DoWork(object sender, DoWorkEventArgs e)
{
for (int i = 0; i < 10; ++i)
{
int percent = i * 10;
bw.ReportProgress(percent);
Thread.Sleep(1000);
}
}
void bw_ProgressChanged(object sender, ProgressChangedEventArgs e)
{
this.Title = "Working: " + e.ProgressPercentage + "%";
}
void bw_RunWorkerCompleted(object sender, RunWorkerCompletedEventArgs e)
{
this.Title = "Finished";
}
}
}
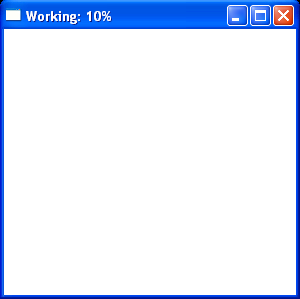