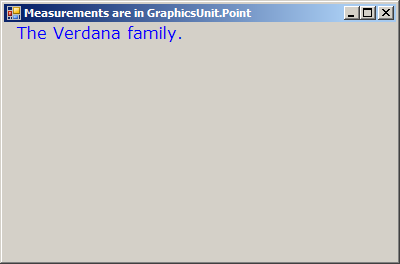
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class FontFamilyAscentDescentLineHeight : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
public FontFamilyAscentDescentLineHeight()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(392, 237);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.OnPaint);
}
[STAThread]
static void Main()
{
Application.Run(new FontFamilyAscentDescentLineHeight());
}
protected void OnPaint (object sender, System.Windows.Forms.PaintEventArgs e)
{
Graphics g = e.Graphics;
FontFamily myFamily = new FontFamily("Verdana");
Font myFont = new Font(myFamily, 12);
int y = 0;
int fontHeight = myFont.Height;
this.Text = "Measurements are in GraphicsUnit." + myFont.Unit.ToString();
g.DrawString("The Verdana family.", myFont, Brushes.Blue, 10, y);
Console.WriteLine("Ascent for bold Verdana: " + myFamily.GetCellAscent(FontStyle.Bold));
Console.WriteLine("Descent for bold Verdana: " + myFamily.GetCellDescent(FontStyle.Bold));
Console.WriteLine("Line spacing for bold Verdana: " + myFamily.GetLineSpacing(FontStyle.Bold));
Console.WriteLine("Height for bold Verdana: " + myFamily.GetEmHeight(FontStyle.Bold));
}
}