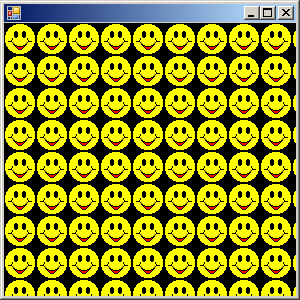
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
public class RectangleTextureFill : System.Windows.Forms.Form
{
private System.ComponentModel.Container components = null;
private Brush brush;
public RectangleTextureFill()
{
InitializeComponent();
try
{
Image img = new Bitmap("YourFile.bmp");
brush = new TextureBrush(img);
}
catch(Exception e)
{ MessageBox.Show(e.Message);}
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(292, 273);
this.Resize += new System.EventHandler(this.RectangleTextureFill_Resize);
this.Paint += new System.Windows.Forms.PaintEventHandler(this.RectangleTextureFill_Paint);
}
[STAThread]
static void Main()
{
Application.Run(new RectangleTextureFill());
}
private void RectangleTextureFill_Resize (object sender, System.EventArgs e)
{
Invalidate();
}
private void RectangleTextureFill_Paint (object sender, PaintEventArgs e)
{
Graphics g = e.Graphics;
Rectangle r = ClientRectangle;
g.FillRectangle(brush, r);
}
}