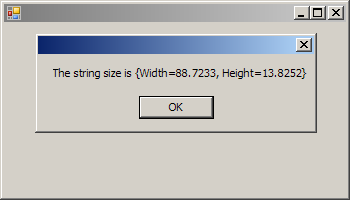
using System;
using System.Drawing;
using System.Windows.Forms;
public class StringMeasure : Form
{
SizeF sz = new SizeF();
public StringMeasure()
{
Size = new Size(350,200);
Button btn = new Button();
btn.Parent = this;
btn.Text = "&Measure";
btn.Location = new Point((ClientSize.Width / 2) - (btn.Width / 2), ClientSize.Height / 2);
btn.Click += new EventHandler(btn_Click);
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
string str = "www.java2s.com";
PointF pt = new PointF(ClientSize.Width / 2, 50);
Graphics g = e.Graphics;
StringFormat fmt = new StringFormat();
fmt.Alignment = StringAlignment.Center;
Brush b = new SolidBrush(ForeColor);
g.DrawString(str, Font, b, pt, fmt);
sz = g.MeasureString(str,Font, pt, fmt);
}
private void btn_Click(object sender, EventArgs e)
{
MessageBox.Show("The string size is " + sz.ToString());
}
static void Main()
{
Application.Run(new StringMeasure());
}
}
27.14.Measure String |
| 27.14.1. | Measure String | 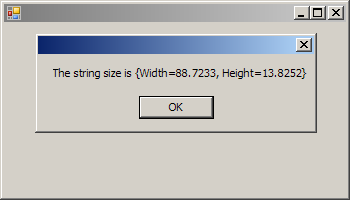 |