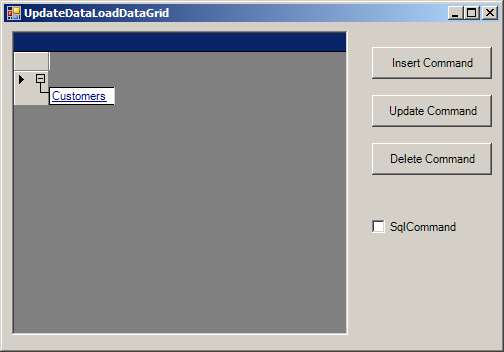
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
using System.Data;
using System.Data.OleDb;
using System.Data.SqlClient;
public class UpdateDataLoadDataGrid : System.Windows.Forms.Form
{
private System.Windows.Forms.DataGrid dataGrid1;
private System.Windows.Forms.Button InsertCommand;
private System.Windows.Forms.Button UpdateCommand;
private System.Windows.Forms.Button DeleteCommand;
private System.Windows.Forms.CheckBox checkBox1;
private System.ComponentModel.Container components = null;
public UpdateDataLoadDataGrid()
{
InitializeComponent();
}
protected override void Dispose( bool disposing )
{
if( disposing )
{
if (components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
private void InitializeComponent()
{
this.dataGrid1 = new System.Windows.Forms.DataGrid();
this.InsertCommand = new System.Windows.Forms.Button();
this.UpdateCommand = new System.Windows.Forms.Button();
this.DeleteCommand = new System.Windows.Forms.Button();
this.checkBox1 = new System.Windows.Forms.CheckBox();
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).BeginInit();
this.SuspendLayout();
//
// dataGrid1
//
this.dataGrid1.DataMember = "";
this.dataGrid1.HeaderForeColor = System.Drawing.SystemColors.ControlText;
this.dataGrid1.Location = new System.Drawing.Point(8, 8);
this.dataGrid1.Name = "dataGrid1";
this.dataGrid1.Size = new System.Drawing.Size(336, 304);
this.dataGrid1.TabIndex = 0;
//
// InsertCommand
//
this.InsertCommand.Location = new System.Drawing.Point(368, 24);
this.InsertCommand.Name = "InsertCommand";
this.InsertCommand.Size = new System.Drawing.Size(120, 32);
this.InsertCommand.TabIndex = 1;
this.InsertCommand.Text = "Insert Command";
this.InsertCommand.Click += new System.EventHandler(this.InsertCommand_Click);
//
// UpdateCommand
//
this.UpdateCommand.Location = new System.Drawing.Point(368, 72);
this.UpdateCommand.Name = "UpdateCommand";
this.UpdateCommand.Size = new System.Drawing.Size(120, 32);
this.UpdateCommand.TabIndex = 2;
this.UpdateCommand.Text = "Update Command";
this.UpdateCommand.Click += new System.EventHandler(this.UpdateCommand_Click);
//
// DeleteCommand
//
this.DeleteCommand.Location = new System.Drawing.Point(368, 120);
this.DeleteCommand.Name = "DeleteCommand";
this.DeleteCommand.Size = new System.Drawing.Size(120, 32);
this.DeleteCommand.TabIndex = 3;
this.DeleteCommand.Text = "Delete Command";
this.DeleteCommand.Click += new System.EventHandler(this.DeleteCommand_Click);
//
// checkBox1
//
this.checkBox1.Location = new System.Drawing.Point(368, 192);
this.checkBox1.Name = "checkBox1";
this.checkBox1.Size = new System.Drawing.Size(112, 24);
this.checkBox1.TabIndex = 4;
this.checkBox1.Text = "SqlCommand";
//
// UpdateDataLoadDataGrid
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 13);
this.ClientSize = new System.Drawing.Size(496, 325);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.checkBox1,
this.DeleteCommand,
this.UpdateCommand,
this.InsertCommand,
this.dataGrid1});
this.Name = "UpdateDataLoadDataGrid";
this.Text = "UpdateDataLoadDataGrid";
((System.ComponentModel.ISupportInitialize)(this.dataGrid1)).EndInit();
this.ResumeLayout(false);
}
[STAThread]
static void Main()
{
Application.Run(new UpdateDataLoadDataGrid());
}
private void InsertCommand_Click(object sender, System.EventArgs e)
{
}
private void UpdateCommand_Click(object sender, System.EventArgs e)
{
string ConnectionString = "Provider=Microsoft.Jet.OLEDB.4.0;"+
"Data Source=c:\\Northwind.mdb";
OleDbConnection conn =
new OleDbConnection(ConnectionString);
DataSet ds = new DataSet();
try
{
conn.Open();
OleDbDataAdapter adapter = new OleDbDataAdapter(
"SELECT * FROM Customers", conn);
OleDbCommandBuilder cmdBuilder =
new OleDbCommandBuilder(adapter);
adapter.MissingSchemaAction =
MissingSchemaAction.AddWithKey;
adapter.Fill(ds, "Customers");
DataRow row1 = ds.Tables["Customers"].Rows.Find("001");
row1["ContactName"]="S";
row1["CompanyName"] = "M";
adapter.Update(ds, "Customers");
dataGrid1.DataSource = ds.DefaultViewManager;
}
catch(OleDbException exp)
{
MessageBox.Show(exp.Message.ToString());
}
if(conn.State == ConnectionState.Open)
conn.Close();
}
private void DeleteCommand_Click(object sender, System.EventArgs e)
{
}
}