The components that supported HTML:
- JButton,
- JLabel,
- JMenuItem,
- JMenu,
- JRadioButtonMenuItem,
- JCheckBoxMenuItem,
- JTabbedPane,
- JToolTip,
- JToggleButton,
- JCheckBox,
- JRadioButton.
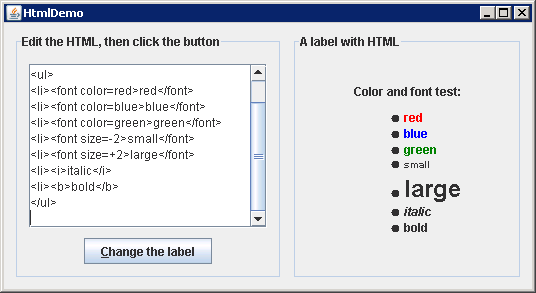
/*
*
* Copyright (c) 1998 Sun Microsystems, Inc. All Rights Reserved.
*
* Sun grants you ("Licensee") a non-exclusive, royalty free, license to use,
* modify and redistribute this software in source and binary code form,
* provided that i) this copyright notice and license appear on all copies of
* the software; and ii) Licensee does not utilize the software in a manner
* which is disparaging to Sun.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY
* IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR
* NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN AND ITS LICENSORS SHALL NOT BE
* LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING
* OR DISTRIBUTING THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL SUN OR ITS
* LICENSORS BE LIABLE FOR ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT,
* INDIRECT, SPECIAL, CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER
* CAUSED AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF
* OR INABILITY TO USE SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* This software is not designed or intended for use in on-line control of
* aircraft, air traffic, aircraft navigation or aircraft communications; or in
* the design, construction, operation or maintenance of any nuclear
* facility. Licensee represents and warrants that it will not use or
* redistribute the Software for such purposes.
*/
import java.awt.Component;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import javax.swing.BorderFactory;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.SwingConstants;
/* HtmlDemo.java needs no other files. */
public class HtmlDemo extends JPanel implements ActionListener {
JLabel theLabel;
JTextArea htmlTextArea;
public HtmlDemo() {
setLayout(new BoxLayout(this, BoxLayout.LINE_AXIS));
String initialText = "<html>\n" + "Color and font test:\n" + "<ul>\n"
+ "<li><font color=red>red</font>\n" + "<li><font color=blue>blue</font>\n"
+ "<li><font color=green>green</font>\n" + "<li><font size=-2>small</font>\n"
+ "<li><font size=+2>large</font>\n" + "<li><i>italic</i>\n" + "<li><b>bold</b>\n"
+ "</ul>\n";
htmlTextArea = new JTextArea(10, 20);
htmlTextArea.setText(initialText);
JScrollPane scrollPane = new JScrollPane(htmlTextArea);
JButton changeTheLabel = new JButton("Change the label");
changeTheLabel.setMnemonic(KeyEvent.VK_C);
changeTheLabel.setAlignmentX(Component.CENTER_ALIGNMENT);
changeTheLabel.addActionListener(this);
theLabel = new JLabel(initialText) {
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
public Dimension getMinimumSize() {
return new Dimension(200, 200);
}
public Dimension getMaximumSize() {
return new Dimension(200, 200);
}
};
theLabel.setVerticalAlignment(SwingConstants.CENTER);
theLabel.setHorizontalAlignment(SwingConstants.CENTER);
JPanel leftPanel = new JPanel();
leftPanel.setLayout(new BoxLayout(leftPanel, BoxLayout.PAGE_AXIS));
leftPanel.setBorder(BorderFactory.createCompoundBorder(BorderFactory
.createTitledBorder("Edit the HTML, then click the button"), BorderFactory
.createEmptyBorder(10, 10, 10, 10)));
leftPanel.add(scrollPane);
leftPanel.add(Box.createRigidArea(new Dimension(0, 10)));
leftPanel.add(changeTheLabel);
JPanel rightPanel = new JPanel();
rightPanel.setLayout(new BoxLayout(rightPanel, BoxLayout.PAGE_AXIS));
rightPanel.setBorder(BorderFactory.createCompoundBorder(BorderFactory
.createTitledBorder("A label with HTML"), BorderFactory.createEmptyBorder(10, 10, 10, 10)));
rightPanel.add(theLabel);
setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
add(leftPanel);
add(Box.createRigidArea(new Dimension(10, 0)));
add(rightPanel);
}
// React to the user pushing the Change button.
public void actionPerformed(ActionEvent e) {
theLabel.setText(htmlTextArea.getText());
}
/**
* Create the GUI and show it. For thread safety, this method should be
* invoked from the event-dispatching thread.
*/
private static void createAndShowGUI() {
// Create and set up the window.
JFrame frame = new JFrame("HtmlDemo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create and set up the content pane.
JComponent newContentPane = new HtmlDemo();
newContentPane.setOpaque(true); // content panes must be opaque
frame.setContentPane(newContentPane);
// Display the window.
frame.pack();
frame.setVisible(true);
}
public static void main(String[] args) {
// Schedule a job for the event-dispatching thread:
// creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}