14.51.JTabbedPane |
| 14.51.1. | First usage of JTabbedPane |
| 14.51.2. | Adding and Removing Tabs | 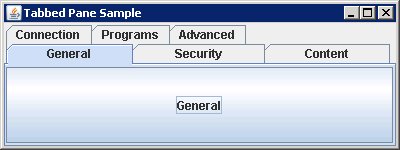 |
| 14.51.3. | Get the index of the first tab that matches an icon |
| 14.51.4. | Changing tab's title, icon, mnemonic, tooltip, or component on a particular tab with one of the setXXXAt() methods | 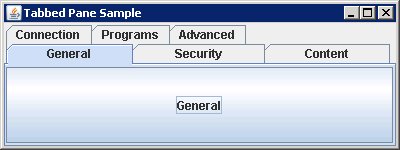 |
| 14.51.5. | Adds tool tips to a table using a renderer |  |
| 14.51.6. | Specifying a tab's location: TOP, BOTTOM, LEFT, or RIGHT | 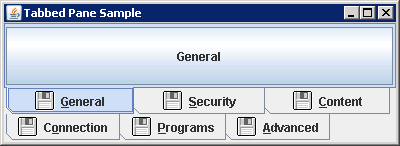 |
| 14.51.7. | Changing background, foreground and icon | 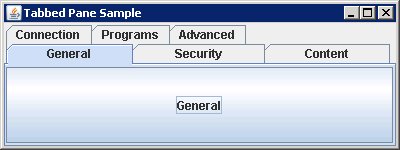 |
| 14.51.8. | To remove a tab, you can remove a specific tab with removeTabAt(int index), remove(int index), or remove(Component component) | 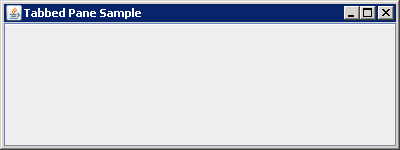 |
| 14.51.9. | Listening for Selected Tab Changes | 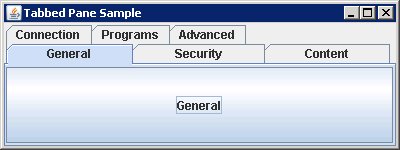 |
| 14.51.10. | TabLayout Policy: SCROLL_TAB_LAYOUT or WRAP_TAP_LAYOUT | 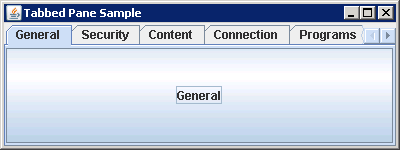 |
| 14.51.11. | Add Components to JTabbedPane |
| 14.51.12. | New Methods in the JTabPane Component (Add component to JTabPane) |
| 14.51.13. | JTabPane with TextField in the tab | 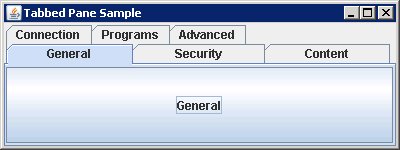 |
| 14.51.14. | Add user icon to tab panel | 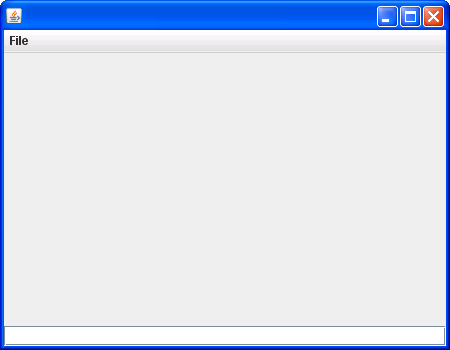 |
| 14.51.15. | Add Button to tab bar | 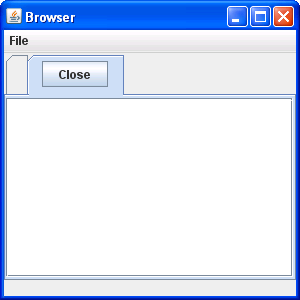 |
| 14.51.16. | Add a tab with a label taken from the name of the component |
| 14.51.17. | Add a tab with a label at the end of all tabs |
| 14.51.18. | Add a tab with a label and icon at the end of all tabs |
| 14.51.19. | Add a tab with a label, icon, and tool tip at the end of all tabs |
| 14.51.20. | Getting the Tabs in a JTabbedPane Container |
| 14.51.21. | Enable Scrolling Tabs in a JTabbedPane Container |
| 14.51.22. | Enabling the Selection of a Tab in a JTabbedPane Container Using a Keystroke |
| 14.51.23. | Enabling and Disabling a Tab in a JTabbedPane Container |
| 14.51.24. | Setting the Color of a Tab in a JTabbedPane Container |
| 14.51.25. | Setting the Tool Tip for a Tab in a JTabbedPane Container |
| 14.51.26. | Setting the Location of the Tabs in a JTabbedPane Container |
| 14.51.27. | Determining When the Selected Tab Changes in a JTabbedPane Container |
| 14.51.28. | Moving a Tab in a JTabbedPane Container |
| 14.51.29. | Insert a tab after the first tab |
| 14.51.30. | Get the index of the tab by matching the child component |
| 14.51.31. | Getting and Setting the Selected Tab in a JTabbedPane Container |
| 14.51.32. | Setting the Size of the Divider in a JSplitPane Container |
| 14.51.33. | Customizing a JTabbedPane Look and Feel |