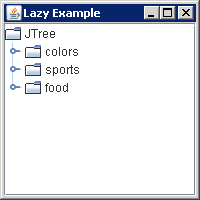
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Component;
import java.awt.Graphics;
import java.awt.Polygon;
import javax.swing.Icon;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTree;
import javax.swing.UIDefaults;
import javax.swing.UIManager;
public class LazySample {
public static void main(String args[]) {
JFrame frame = new JFrame("Lazy Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Integer fifteen = new Integer(15);
Object lazyArgs[] = new Object[] { Color.GREEN, Boolean.TRUE, fifteen, fifteen };
Object lazyDiamond = new UIDefaults.ProxyLazyValue("MyIcon", lazyArgs);
UIManager.put("Tree.openIcon", lazyDiamond);
JTree tree = new JTree();
JScrollPane scrollPane = new JScrollPane(tree);
frame.add(scrollPane, BorderLayout.CENTER);
frame.setSize(200, 200);
frame.setVisible(true);
}
}
class MyIcon implements Icon {
public MyIcon(Object obj) {
}
public int getIconHeight() {
return 20;
}
public int getIconWidth() {
return 20;
}
public void paintIcon(Component c, Graphics g, int x, int y) {
g.setColor(Color.RED);
g.fillRect(1,1,20,20);
}
}