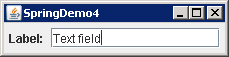
/*
*
* Copyright (c) 1998 Sun Microsystems, Inc. All Rights Reserved.
*
* Sun grants you ("Licensee") a non-exclusive, royalty free, license to use,
* modify and redistribute this software in source and binary code form,
* provided that i) this copyright notice and license appear on all copies of
* the software; and ii) Licensee does not utilize the software in a manner
* which is disparaging to Sun.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY
* IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR
* NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN AND ITS LICENSORS SHALL NOT BE
* LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING
* OR DISTRIBUTING THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL SUN OR ITS
* LICENSORS BE LIABLE FOR ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT,
* INDIRECT, SPECIAL, CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER
* CAUSED AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF
* OR INABILITY TO USE SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* This software is not designed or intended for use in on-line control of
* aircraft, air traffic, aircraft navigation or aircraft communications; or in
* the design, construction, operation or maintenance of any nuclear
* facility. Licensee represents and warrants that it will not use or
* redistribute the Software for such purposes.
*/
import java.awt.Component;
import java.awt.Container;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JTextField;
import javax.swing.Spring;
import javax.swing.SpringLayout;
public class SpringDemo4 {
public static void main(String[] args) {
JFrame frame = new JFrame("SpringDemo4");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Set up the content pane.
Container contentPane = frame.getContentPane();
SpringLayout layout = new SpringLayout();
contentPane.setLayout(layout);
// Create and add the components.
JLabel label = new JLabel("Label: ");
JTextField textField = new JTextField("Text field", 15);
contentPane.add(label);
contentPane.add(textField);
// Adjust constraints for the label so it's at (5,5).
SpringLayout.Constraints labelCons = layout.getConstraints(label);
labelCons.setX(Spring.constant(5));
labelCons.setY(Spring.constant(5));
// Adjust constraints for the text field so it's at
// (<label's right edge> + 5, 5).
SpringLayout.Constraints textFieldCons = layout.getConstraints(textField);
textFieldCons.setX(Spring.sum(Spring.constant(5), labelCons.getConstraint(SpringLayout.EAST)));
textFieldCons.setY(Spring.constant(5));
// Adjust constraints for the content pane.
setContainerSize(contentPane, 5);
// Display the window.
frame.pack();
frame.setVisible(true);
}
public static void setContainerSize(Container parent, int pad) {
SpringLayout layout = (SpringLayout) parent.getLayout();
Component[] components = parent.getComponents();
Spring maxHeightSpring = Spring.constant(0);
SpringLayout.Constraints pCons = layout.getConstraints(parent);
// Set the container's right edge to the right edge
// of its rightmost component + padding.
Component rightmost = components[components.length - 1];
SpringLayout.Constraints rCons = layout.getConstraints(rightmost);
pCons.setConstraint(SpringLayout.EAST, Spring.sum(Spring.constant(pad), rCons
.getConstraint(SpringLayout.EAST)));
// Set the container's bottom edge to the bottom edge
// of its tallest component + padding.
for (int i = 0; i < components.length; i++) {
SpringLayout.Constraints cons = layout.getConstraints(components[i]);
maxHeightSpring = Spring.max(maxHeightSpring, cons.getConstraint(SpringLayout.SOUTH));
}
pCons.setConstraint(SpringLayout.SOUTH, Spring.sum(Spring.constant(pad), maxHeightSpring));
}
}