TableRowSorter without column class : JTable Sort « Swing « Java Tutorial
- Java Tutorial
- Swing
- JTable Sort
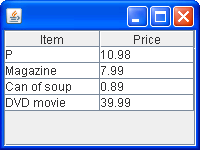
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.RowSorter;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import javax.swing.table.TableRowSorter;
public class TableRowSorterWithoutColumnClass extends JFrame {
public TableRowSorterWithoutColumnClass() {
setDefaultCloseOperation(EXIT_ON_CLOSE);
String[] columns = { "Item", "Price" };
Object[][] rows = { { "P", 10.98 }, { "Magazine", 7.99 },
{ "Can of soup", 0.89 }, { "DVD movie", 39.99 } };
TableModel model = new DefaultTableModel(rows, columns);
JTable table = new JTable(model);
RowSorter<TableModel> sorter = new TableRowSorter<TableModel>(model);
table.setRowSorter(sorter);
getContentPane().add(new JScrollPane(table));
setSize(200, 150);
setVisible(true);
}
public static void main(String[] args) {
new TableRowSorterWithoutColumnClass();
}
}