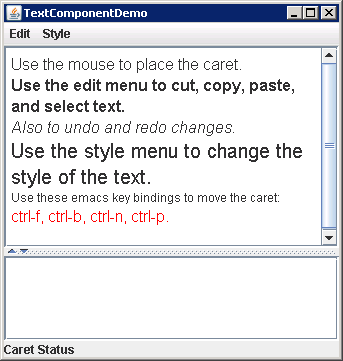
/*
*
* Copyright (c) 1998 Sun Microsystems, Inc. All Rights Reserved.
*
* Sun grants you ("Licensee") a non-exclusive, royalty free, license to use,
* modify and redistribute this software in source and binary code form,
* provided that i) this copyright notice and license appear on all copies of
* the software; and ii) Licensee does not utilize the software in a manner
* which is disparaging to Sun.
*
* This software is provided "AS IS," without a warranty of any kind. ALL
* EXPRESS OR IMPLIED CONDITIONS, REPRESENTATIONS AND WARRANTIES, INCLUDING ANY
* IMPLIED WARRANTY OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE OR
* NON-INFRINGEMENT, ARE HEREBY EXCLUDED. SUN AND ITS LICENSORS SHALL NOT BE
* LIABLE FOR ANY DAMAGES SUFFERED BY LICENSEE AS A RESULT OF USING, MODIFYING
* OR DISTRIBUTING THE SOFTWARE OR ITS DERIVATIVES. IN NO EVENT WILL SUN OR ITS
* LICENSORS BE LIABLE FOR ANY LOST REVENUE, PROFIT OR DATA, OR FOR DIRECT,
* INDIRECT, SPECIAL, CONSEQUENTIAL, INCIDENTAL OR PUNITIVE DAMAGES, HOWEVER
* CAUSED AND REGARDLESS OF THE THEORY OF LIABILITY, ARISING OUT OF THE USE OF
* OR INABILITY TO USE SOFTWARE, EVEN IF SUN HAS BEEN ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGES.
*
* This software is not designed or intended for use in on-line control of
* aircraft, air traffic, aircraft navigation or aircraft communications; or in
* the design, construction, operation or maintenance of any nuclear
* facility. Licensee represents and warrants that it will not use or
* redistribute the Software for such purposes.
*/
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Event;
import java.awt.GridLayout;
import java.awt.Insets;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.KeyEvent;
import java.util.HashMap;
import javax.swing.AbstractAction;
import javax.swing.Action;
import javax.swing.InputMap;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.JTextArea;
import javax.swing.JTextPane;
import javax.swing.KeyStroke;
import javax.swing.SwingUtilities;
import javax.swing.event.CaretEvent;
import javax.swing.event.CaretListener;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import javax.swing.event.UndoableEditEvent;
import javax.swing.event.UndoableEditListener;
import javax.swing.text.AbstractDocument;
import javax.swing.text.BadLocationException;
import javax.swing.text.DefaultEditorKit;
import javax.swing.text.Document;
import javax.swing.text.JTextComponent;
import javax.swing.text.SimpleAttributeSet;
import javax.swing.text.StyleConstants;
import javax.swing.text.StyledDocument;
import javax.swing.text.StyledEditorKit;
import javax.swing.undo.CannotRedoException;
import javax.swing.undo.CannotUndoException;
import javax.swing.undo.UndoManager;
public class TextComponentDemo extends JFrame {
JTextPane textPane;
AbstractDocument doc;
static final int MAX_CHARACTERS = 300;
JTextArea changeLog;
String newline = "\n";
HashMap<Object, Action> actions;
// undo helpers
protected UndoAction undoAction;
protected RedoAction redoAction;
protected UndoManager undo = new UndoManager();
public TextComponentDemo() {
super("TextComponentDemo");
// Create the text pane and configure it.
textPane = new JTextPane();
textPane.setCaretPosition(0);
textPane.setMargin(new Insets(5, 5, 5, 5));
StyledDocument styledDoc = textPane.getStyledDocument();
if (styledDoc instanceof AbstractDocument) {
doc = (AbstractDocument) styledDoc;
//doc.setDocumentFilter(new DocumentSizeFilter(MAX_CHARACTERS));
} else {
System.err.println("Text pane's document isn't an AbstractDocument!");
System.exit(-1);
}
JScrollPane scrollPane = new JScrollPane(textPane);
scrollPane.setPreferredSize(new Dimension(200, 200));
// Create the text area for the status log and configure it.
changeLog = new JTextArea(5, 30);
changeLog.setEditable(false);
JScrollPane scrollPaneForLog = new JScrollPane(changeLog);
// Create a split pane for the change log and the text area.
JSplitPane splitPane = new JSplitPane(JSplitPane.VERTICAL_SPLIT, scrollPane, scrollPaneForLog);
splitPane.setOneTouchExpandable(true);
// Create the status area.
JPanel statusPane = new JPanel(new GridLayout(1, 1));
CaretListenerLabel caretListenerLabel = new CaretListenerLabel("Caret Status");
statusPane.add(caretListenerLabel);
// Add the components.
getContentPane().add(splitPane, BorderLayout.CENTER);
getContentPane().add(statusPane, BorderLayout.PAGE_END);
// Set up the menu bar.
createActionTable(textPane);
JMenu editMenu = createEditMenu();
JMenu styleMenu = createStyleMenu();
JMenuBar mb = new JMenuBar();
mb.add(editMenu);
mb.add(styleMenu);
setJMenuBar(mb);
// Add some key bindings.
addBindings();
// Put the initial text into the text pane.
initDocument();
// Start watching for undoable edits and caret changes.
doc.addUndoableEditListener(new MyUndoableEditListener());
textPane.addCaretListener(caretListenerLabel);
doc.addDocumentListener(new MyDocumentListener());
}
// This listens for and reports caret movements.
protected class CaretListenerLabel extends JLabel implements CaretListener {
public CaretListenerLabel(String label) {
super(label);
}
// Might not be invoked from the event dispatching thread.
public void caretUpdate(CaretEvent e) {
displaySelectionInfo(e.getDot(), e.getMark());
}
protected void displaySelectionInfo(final int dot, final int mark) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
if (dot == mark) { // no selection
try {
Rectangle caretCoords = textPane.modelToView(dot);
// Convert it to view coordinates.
setText("caret: text position: " + dot + ", view location = [" + caretCoords.x + ", "
+ caretCoords.y + "]" + newline);
} catch (BadLocationException ble) {
setText("caret: text position: " + dot + newline);
}
} else if (dot < mark) {
setText("selection from: " + dot + " to " + mark + newline);
} else {
setText("selection from: " + mark + " to " + dot + newline);
}
}
});
}
}
// This one listens for edits that can be undone.
protected class MyUndoableEditListener implements UndoableEditListener {
public void undoableEditHappened(UndoableEditEvent e) {
// Remember the edit and update the menus.
undo.addEdit(e.getEdit());
undoAction.updateUndoState();
redoAction.updateRedoState();
}
}
// And this one listens for any changes to the document.
protected class MyDocumentListener implements DocumentListener {
public void insertUpdate(DocumentEvent e) {
displayEditInfo(e);
}
public void removeUpdate(DocumentEvent e) {
displayEditInfo(e);
}
public void changedUpdate(DocumentEvent e) {
displayEditInfo(e);
}
private void displayEditInfo(DocumentEvent e) {
Document document = (Document) e.getDocument();
int changeLength = e.getLength();
changeLog.append(e.getType().toString() + ": " + changeLength + " character"
+ ((changeLength == 1) ? ". " : "s. ") + " Text length = " + document.getLength() + "."
+ newline);
}
}
// Add a couple of emacs key bindings for navigation.
protected void addBindings() {
InputMap inputMap = textPane.getInputMap();
// Ctrl-b to go backward one character
KeyStroke key = KeyStroke.getKeyStroke(KeyEvent.VK_B, Event.CTRL_MASK);
inputMap.put(key, DefaultEditorKit.backwardAction);
// Ctrl-f to go forward one character
key = KeyStroke.getKeyStroke(KeyEvent.VK_F, Event.CTRL_MASK);
inputMap.put(key, DefaultEditorKit.forwardAction);
// Ctrl-p to go up one line
key = KeyStroke.getKeyStroke(KeyEvent.VK_P, Event.CTRL_MASK);
inputMap.put(key, DefaultEditorKit.upAction);
// Ctrl-n to go down one line
key = KeyStroke.getKeyStroke(KeyEvent.VK_N, Event.CTRL_MASK);
inputMap.put(key, DefaultEditorKit.downAction);
}
// Create the edit menu.
protected JMenu createEditMenu() {
JMenu menu = new JMenu("Edit");
// Undo and redo are actions of our own creation.
undoAction = new UndoAction();
menu.add(undoAction);
redoAction = new RedoAction();
menu.add(redoAction);
menu.addSeparator();
// These actions come from the default editor kit.
// Get the ones we want and stick them in the menu.
menu.add(getActionByName(DefaultEditorKit.cutAction));
menu.add(getActionByName(DefaultEditorKit.copyAction));
menu.add(getActionByName(DefaultEditorKit.pasteAction));
menu.addSeparator();
menu.add(getActionByName(DefaultEditorKit.selectAllAction));
return menu;
}
// Create the style menu.
protected JMenu createStyleMenu() {
JMenu menu = new JMenu("Style");
Action action = new StyledEditorKit.BoldAction();
action.putValue(Action.NAME, "Bold");
menu.add(action);
action = new StyledEditorKit.ItalicAction();
action.putValue(Action.NAME, "Italic");
menu.add(action);
action = new StyledEditorKit.UnderlineAction();
action.putValue(Action.NAME, "Underline");
menu.add(action);
menu.addSeparator();
menu.add(new StyledEditorKit.FontSizeAction("12", 12));
menu.add(new StyledEditorKit.FontSizeAction("14", 14));
menu.add(new StyledEditorKit.FontSizeAction("18", 18));
menu.addSeparator();
menu.add(new StyledEditorKit.FontFamilyAction("Serif", "Serif"));
menu.add(new StyledEditorKit.FontFamilyAction("SansSerif", "SansSerif"));
menu.addSeparator();
menu.add(new StyledEditorKit.ForegroundAction("Red", Color.red));
menu.add(new StyledEditorKit.ForegroundAction("Green", Color.green));
menu.add(new StyledEditorKit.ForegroundAction("Blue", Color.blue));
menu.add(new StyledEditorKit.ForegroundAction("Black", Color.black));
return menu;
}
protected void initDocument() {
String initString[] = { "Use the mouse to place the caret.",
"Use the edit menu to cut, copy, paste, and select text.",
"Also to undo and redo changes.", "Use the style menu to change the style of the text.",
"Use these emacs key bindings to move the caret:", "ctrl-f, ctrl-b, ctrl-n, ctrl-p." };
SimpleAttributeSet[] attrs = initAttributes(initString.length);
try {
for (int i = 0; i < initString.length; i++) {
doc.insertString(doc.getLength(), initString[i] + newline, attrs[i]);
}
} catch (BadLocationException ble) {
System.err.println("Couldn't insert initial text.");
}
}
protected SimpleAttributeSet[] initAttributes(int length) {
// Hard-code some attributes.
SimpleAttributeSet[] attrs = new SimpleAttributeSet[length];
attrs[0] = new SimpleAttributeSet();
StyleConstants.setFontFamily(attrs[0], "SansSerif");
StyleConstants.setFontSize(attrs[0], 16);
attrs[1] = new SimpleAttributeSet(attrs[0]);
StyleConstants.setBold(attrs[1], true);
attrs[2] = new SimpleAttributeSet(attrs[0]);
StyleConstants.setItalic(attrs[2], true);
attrs[3] = new SimpleAttributeSet(attrs[0]);
StyleConstants.setFontSize(attrs[3], 20);
attrs[4] = new SimpleAttributeSet(attrs[0]);
StyleConstants.setFontSize(attrs[4], 12);
attrs[5] = new SimpleAttributeSet(attrs[0]);
StyleConstants.setForeground(attrs[5], Color.red);
return attrs;
}
// The following two methods allow us to find an
// action provided by the editor kit by its name.
private void createActionTable(JTextComponent textComponent) {
actions = new HashMap<Object, Action>();
Action[] actionsArray = textComponent.getActions();
for (int i = 0; i < actionsArray.length; i++) {
Action a = actionsArray[i];
actions.put(a.getValue(Action.NAME), a);
}
}
private Action getActionByName(String name) {
return actions.get(name);
}
class UndoAction extends AbstractAction {
public UndoAction() {
super("Undo");
setEnabled(false);
}
public void actionPerformed(ActionEvent e) {
try {
undo.undo();
} catch (CannotUndoException ex) {
System.out.println("Unable to undo: " + ex);
ex.printStackTrace();
}
updateUndoState();
redoAction.updateRedoState();
}
protected void updateUndoState() {
if (undo.canUndo()) {
setEnabled(true);
putValue(Action.NAME, undo.getUndoPresentationName());
} else {
setEnabled(false);
putValue(Action.NAME, "Undo");
}
}
}
class RedoAction extends AbstractAction {
public RedoAction() {
super("Redo");
setEnabled(false);
}
public void actionPerformed(ActionEvent e) {
try {
undo.redo();
} catch (CannotRedoException ex) {
System.out.println("Unable to redo: " + ex);
ex.printStackTrace();
}
updateRedoState();
undoAction.updateUndoState();
}
protected void updateRedoState() {
if (undo.canRedo()) {
setEnabled(true);
putValue(Action.NAME, undo.getRedoPresentationName());
} else {
setEnabled(false);
putValue(Action.NAME, "Redo");
}
}
}
/**
* Create the GUI and show it. For thread safety, this method should be
* invoked from the event-dispatching thread.
*/
private static void createAndShowGUI() {
// Create and set up the window.
final TextComponentDemo frame = new TextComponentDemo();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Display the window.
frame.pack();
frame.setVisible(true);
}
// The standard main method.
public static void main(String[] args) {
// Schedule a job for the event-dispatching thread:
// creating and showing this application's GUI.
javax.swing.SwingUtilities.invokeLater(new Runnable() {
public void run() {
createAndShowGUI();
}
});
}
}