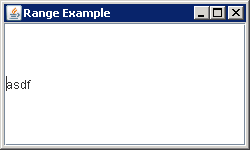
import javax.swing.JFrame;
import javax.swing.JTextField;
import javax.swing.text.AbstractDocument;
import javax.swing.text.AttributeSet;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import javax.swing.text.DocumentFilter;
class IntegerRangeDocumentFilter extends DocumentFilter {
public IntegerRangeDocumentFilter() {
}
public void insertString(DocumentFilter.FilterBypass fb, int offset, String string,
AttributeSet attr) throws BadLocationException {
System.out.println("insert string"+ string);
System.out.println(offset);
super.insertString(fb, offset, string, attr);
}
public void remove(DocumentFilter.FilterBypass fb, int offset, int length)
throws BadLocationException {
System.out.println("remove");
super.remove(fb, offset, length);
}
public void replace(DocumentFilter.FilterBypass fb, int offset, int length, String text,
AttributeSet attrs) throws BadLocationException {
System.out.println("replace");
super.replace(fb, offset, length, text, attrs);
}
}
public class RangeSample {
public static void main(String args[]) {
JFrame frame = new JFrame("Range Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JTextField textFieldOne = new JTextField();
Document textDocOne = textFieldOne.getDocument();
DocumentFilter filterOne = new IntegerRangeDocumentFilter();
((AbstractDocument) textDocOne).setDocumentFilter(filterOne);
frame.add(textFieldOne);
frame.setSize(250, 150);
frame.setVisible(true);
}
}