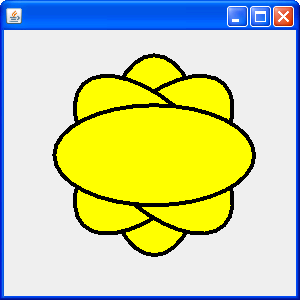
import java.awt.BasicStroke;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import javax.swing.JComponent;
import javax.swing.JFrame;
public class RotateStrokeEllipse extends JFrame {
public static void main(String[] args) {
new RotateStrokeEllipse();
}
public RotateStrokeEllipse() {
this.setSize(300, 300);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.add(new PaintSurface(), BorderLayout.CENTER);
this.setVisible(true);
}
private class PaintSurface extends JComponent {
public PaintSurface() {
}
public void paint(Graphics g) {
Graphics2D g2 = (Graphics2D) g;
int x = 50;
int y = 75;
int width = 200;
int height = 100;
Shape r1 = new Ellipse2D.Float(x, y, width, height);
for (int angle = 0; angle <= 360; angle += 45) {
g2.rotate(Math.toRadians(angle), x + width / 2, y + height / 2);
g2.setPaint(Color.YELLOW);
g2.fill(r1);
g2.setStroke(new BasicStroke(4));
g2.setPaint(Color.BLACK);
g2.draw(r1);
}
}
}
}