Add Buttons to ToolBar : ToolBar « SWT « Java Tutorial
- Java Tutorial
- SWT
- ToolBar
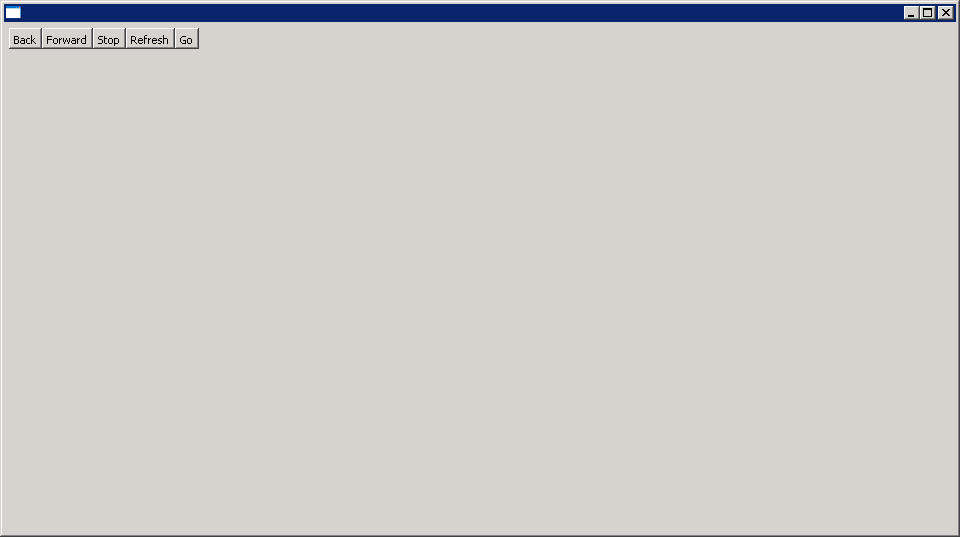
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.ToolBar;
import org.eclipse.swt.widgets.ToolItem;
public class ToolBarButtonsAdding {
public static void main(String[] args) {
Display display = new Display();
final Shell shell = new Shell(display);
GridLayout gridLayout = new GridLayout();
gridLayout.numColumns = 3;
shell.setLayout(gridLayout);
ToolBar toolbar = new ToolBar(shell, SWT.NONE);
ToolItem itemBack = new ToolItem(toolbar, SWT.PUSH);
itemBack.setText("Back");
ToolItem itemForward = new ToolItem(toolbar, SWT.PUSH);
itemForward.setText("Forward");
ToolItem itemStop = new ToolItem(toolbar, SWT.PUSH);
itemStop.setText("Stop");
ToolItem itemRefresh = new ToolItem(toolbar, SWT.PUSH);
itemRefresh.setText("Refresh");
ToolItem itemGo = new ToolItem(toolbar, SWT.PUSH);
itemGo.setText("Go");
GridData data = new GridData();
data.horizontalSpan = 3;
toolbar.setLayoutData(data);
Listener listener = new Listener() {
public void handleEvent(Event event) {
ToolItem item = (ToolItem) event.widget;
String string = item.getText();
if (string.equals("Back"))
System.out.println("Back");
else if (string.equals("Forward"))
System.out.println("Forward");
else if (string.equals("Stop"))
System.out.println("Stop");
else if (string.equals("Refresh"))
System.out.println("Refresh");
else if (string.equals("Go"))
System.out.println("Go");
}
};
itemBack.addListener(SWT.Selection, listener);
itemForward.addListener(SWT.Selection, listener);
itemStop.addListener(SWT.Selection, listener);
itemRefresh.addListener(SWT.Selection, listener);
itemGo.addListener(SWT.Selection, listener);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}