The TableColumn class represents a column in the table.
You create a column with a parent table, a style, and optionally an index.
If you don't specify an index, the column assumes the next available zero-based index.
public TableColumn(Table parent, int style)
public TableColumn(Table parent, int style, int index)
The supported styles all specify the alignment for the column's contents:
- SWT.LEFT for left alignment,
- SWT.CENTER for center alignment, and
- SWT.RIGHT for right alignment.
- You should specify only one of these;
- You can change alignment after construction using the setAlignment() method.
- Alignment defaults to left and affects all rows in the column.
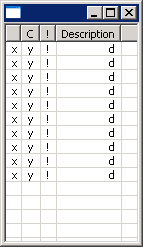
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Table;
import org.eclipse.swt.widgets.TableColumn;
import org.eclipse.swt.widgets.TableItem;
public class TableColumnAdding {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
Table table = new Table(shell, SWT.MULTI | SWT.BORDER | SWT.FULL_SELECTION);
table.setLinesVisible(true);
table.setHeaderVisible(true);
String[] titles = { " ", "C", "!", "Description"};
for (int i = 0; i < titles.length; i++) {
TableColumn column = new TableColumn(table, SWT.RIGHT);
column.setText(titles[i]);
}
for (int i = 0; i < 10; i++) {
TableItem item = new TableItem(table, SWT.NONE);
item.setText(0, "x");
item.setText(1, "y");
item.setText(2, "!");
item.setText(3, "d");
}
for (int i=0; i<titles.length; i++) {
table.getColumn (i).pack ();
}
table.setSize(table.computeSize(SWT.DEFAULT, 200));
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}