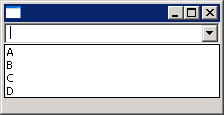
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Combo;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class CreateDropCombo {
// Strings to use as list items
private static final String[] ITEMS = { "A", "B", "C", "D" };
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setLayout(new FillLayout());
// Create a dropdown Combo
Combo combo = new Combo(shell, SWT.DROP_DOWN);
combo.setItems(ITEMS);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}