int altEAction = styledText.getKeyBinding('e' | SWT.ALT);
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.StyledText;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class StyledTextActionBound {
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display);
StyledText styledText = new StyledText(shell, SWT.V_SCROLL | SWT.BORDER);
styledText.setText("12345");
int altEAction = styledText.getKeyBinding('e' | SWT.ALT);
styledText.setBounds(10, 10, 100, 100);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
To get the action for Shift+Left, use this code:
int shiftLeftAction = styledText.getKeyBinding(SWT.ARROW_LEFT | SWT.SHIFT);
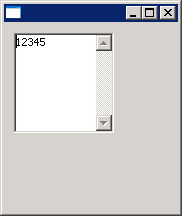
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.StyledText;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class StyledTextActionBoundArrowShift {
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display);
StyledText styledText = new StyledText(shell, SWT.V_SCROLL | SWT.BORDER);
styledText.setText("12345");
int shiftLeftAction = styledText.getKeyBinding(SWT.ARROW_LEFT | SWT.SHIFT);
styledText.setBounds(10, 10, 100, 100);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}