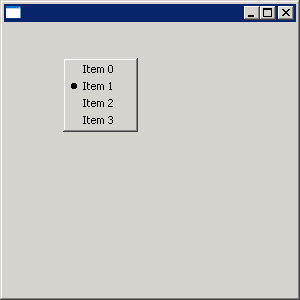
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
public class MenuItemPopupRadio {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
Menu menu = new Menu(shell, SWT.POP_UP);
for (int i = 0; i < 4; i++) {
MenuItem item = new MenuItem(menu, SWT.RADIO);
item.setText("Item " + i);
item.addSelectionListener(new SelectionAdapter() {
public void widgetSelected(SelectionEvent e) {
MenuItem item = (MenuItem) e.widget;
if (item.getSelection()) {
System.out.println(item + " selected");
} else {
System.out.println(item + " unselected");
}
}
});
}
shell.setMenu(menu);
shell.setSize(300, 300);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
display.dispose();
}
}