Select a range : List « SWT « Java Tutorial
- Java Tutorial
- SWT
- List
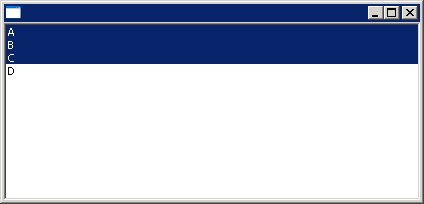
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.List;
import org.eclipse.swt.widgets.Shell;
public class SelectRangeList {
// Strings to use as list items
private static final String[] ITEMS = { "A", "B", "C", "D" };
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setLayout(new FillLayout());
// Create a multiple-selection list
List multi = new List(shell, SWT.BORDER | SWT.MULTI | SWT.V_SCROLL);
// Add the items all at once
multi.setItems(ITEMS);
// Select a range
multi.select(0, 2);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}