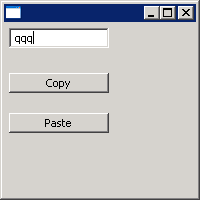
import org.eclipse.swt.SWT;
import org.eclipse.swt.dnd.Clipboard;
import org.eclipse.swt.dnd.TextTransfer;
import org.eclipse.swt.dnd.Transfer;
import org.eclipse.swt.widgets.Button;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.Text;
public class ClipBoardCopyPaster {
public static void main( String[] args) {
Display display = new Display ();
final Clipboard cb = new Clipboard(display);
final Shell shell = new Shell (display);
final Text text = new Text(shell, SWT.BORDER | SWT.SINGLE);
text.setBounds(5,5,100,20);
Button copy = new Button(shell, SWT.PUSH);
copy.setBounds(5,50,100,20);
copy.setText("Copy");
copy.addListener (SWT.Selection, new Listener () {
public void handleEvent (Event e) {
String textData = text.getSelectionText();
if (textData.length() > 0) {
TextTransfer textTransfer = TextTransfer.getInstance();
cb.setContents(new Object[]{textData}, new Transfer[]{textTransfer});
}
}
});
Button paste = new Button(shell, SWT.PUSH);
paste.setBounds(5,90,100,20);
paste.setText("Paste");
paste.addListener (SWT.Selection, new Listener () {
public void handleEvent (Event e) {
TextTransfer transfer = TextTransfer.getInstance();
String data = (String)cb.getContents(transfer);
if (data != null) {
text.insert(data);
}
}
});
shell.setSize(200, 200);
shell.open();
while (!shell.isDisposed ()) {
if (!display.readAndDispatch ()) display.sleep ();
}
cb.dispose();
display.dispose();
}
}