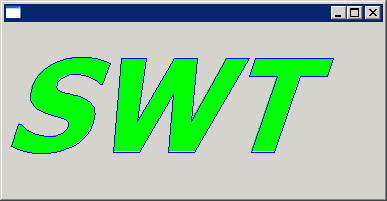
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.FontData;
import org.eclipse.swt.graphics.GC;
import org.eclipse.swt.graphics.Path;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Shell;
public class PathTextFrom {
public static void main(String[] args) {
Display display = new Display();
FontData data = display.getSystemFont().getFontData()[0];
Font font = new Font(display, data.getName(), 96, SWT.BOLD | SWT.ITALIC);
final Color green = display.getSystemColor(SWT.COLOR_GREEN);
final Color blue = display.getSystemColor(SWT.COLOR_BLUE);
final Path path = new Path(display);
path.addString("SWT", 0, 0, font);
Shell shell = new Shell(display);
shell.addListener(SWT.Paint, new Listener() {
public void handleEvent(Event e) {
GC gc = e.gc;
gc.setBackground(green);
gc.setForeground(blue);
gc.fillPath(path);
gc.drawPath(path);
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch())
display.sleep();
}
path.dispose();
font.dispose();
display.dispose();
}
}