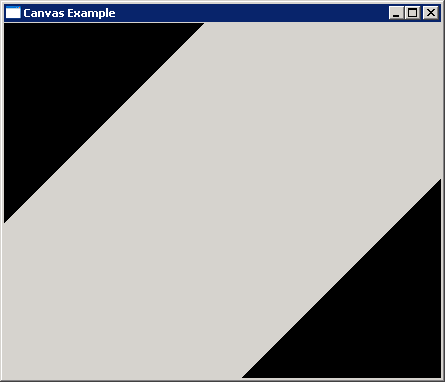
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.PaintEvent;
import org.eclipse.swt.events.PaintListener;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Canvas;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class DrawPolygonSWT {
public static void main(String[] args) {
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("Canvas Example");
shell.setLayout(new FillLayout());
Canvas canvas = new Canvas(shell, SWT.NONE);
canvas.addPaintListener(new PaintListener() {
public void paintControl(PaintEvent e) {
Canvas canvas = (Canvas) e.widget;
int x = canvas.getBounds().width;
int y = canvas.getBounds().height;
e.gc.setBackground(e.display.getSystemColor(SWT.COLOR_BLACK));
// Create the points for drawing a triangle in the upper left
int[] upper_left = { 0, 0, 200, 0, 0, 200};
// Create the points for drawing a triangle in the lower right
int[] lower_right = { x, y, x, y - 200, x - 200, y};
// Draw the triangles
e.gc.fillPolygon(upper_left);
e.gc.fillPolygon(lower_right); }
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
18.11.Polygon |
| 18.11.1. | Drawing Polygons | 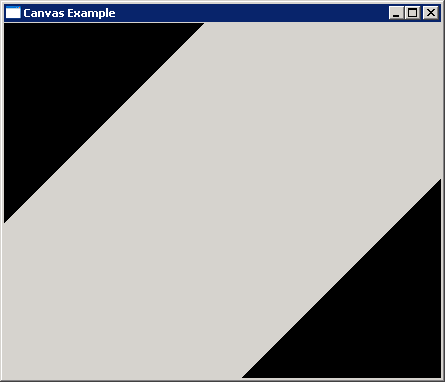 |