Term | Meaning |
baseline | The imaginary line the font sits on |
ascent | The number of pixels that characters reach above the baseline to the top of typical lowercase characters |
descent | The number of pixels that characters reach below the baseline |
height | The total height of characters in pixels, equal to the ascent plus the descent plus the leading area |
leading area | The number of pixels above the top of typical lowercase characters |
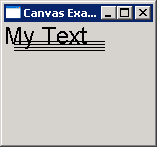
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.PaintEvent;
import org.eclipse.swt.events.PaintListener;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.FontData;
import org.eclipse.swt.graphics.FontMetrics;
import org.eclipse.swt.layout.FillLayout;
import org.eclipse.swt.widgets.Canvas;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
public class FontMetricsPaintSWT {
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display);
shell.setText("Canvas Example");
shell.setLayout(new FillLayout());
Canvas canvas = new Canvas(shell, SWT.NONE);
canvas.addPaintListener(new PaintListener() {
public void paintControl(PaintEvent e) {
Font font = new Font(shell.getDisplay(), new FontData("Helvetica", 18, SWT.NORMAL));
e.gc.setFont(font);
e.gc.drawText("My Text", 0, 0);
FontMetrics fm = e.gc.getFontMetrics();
int bHeight = fm.getLeading() + fm.getAscent();
int oHeight = fm.getAscent();
int yHeight = fm.getAscent() + fm.getDescent();
int totalHeight = fm.getHeight(); // Equals fm.getLeading() + fm.getAscent()
// + fm.getDescent();
e.gc.drawLine(10,bHeight,100,bHeight);
e.gc.drawLine(10,oHeight,100,oHeight);
e.gc.drawLine(10,yHeight,100,yHeight);
e.gc.drawLine(10,totalHeight,100,totalHeight);
font.dispose();
}
});
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}