Add choice to ChoiceGroup : ChoiceGroup « J2ME « Java Tutorial
- Java Tutorial
- J2ME
- ChoiceGroup
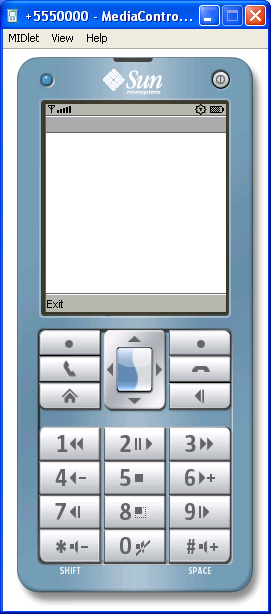
import javax.microedition.lcdui.Choice;
import javax.microedition.lcdui.ChoiceGroup;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.Item;
import javax.microedition.lcdui.ItemStateListener;
import javax.microedition.lcdui.StringItem;
import javax.microedition.midlet.MIDlet;
public class J2MERadioButtons extends MIDlet implements ItemStateListener, CommandListener {
private Display display;
private Form form = new Form("");
private Command exit = new Command("Exit", Command.EXIT, 1);
private ChoiceGroup radioButtons = new ChoiceGroup("Select Your Color", Choice.EXCLUSIVE);
private int defaultIndex;
public J2MERadioButtons() {
display = Display.getDisplay(this);
radioButtons.append("Red", null);
radioButtons.append("White", null);
radioButtons.append("Blue", null);
radioButtons.append("Green", null);
defaultIndex = radioButtons.append("All", null);
radioButtons.setSelectedIndex(defaultIndex, true);
form.addCommand(exit);
form.setCommandListener(this);
form.setItemStateListener(this);
}
public void startApp() {
display.setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command command, Displayable displayable) {
if (command == exit) {
destroyApp(true);
notifyDestroyed();
}
}
public void itemStateChanged(Item item) {
if (item == radioButtons) {
StringItem msg = new StringItem("Your color is ", radioButtons.getString(radioButtons.getSelectedIndex()));
form.append(msg);
}
}
}