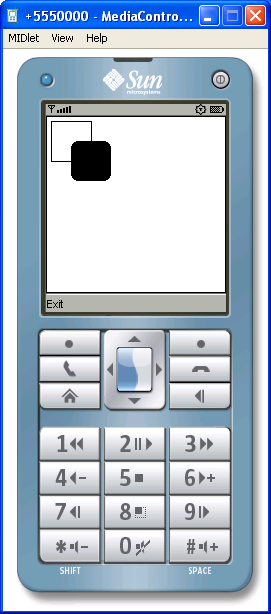
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Graphics;
import javax.microedition.midlet.MIDlet;
public class RectMIDlet extends MIDlet implements CommandListener {
private Command exitCommand;
Display display;
public void startApp() {
Display display = Display.getDisplay(this);
Displayable d = new RectCanvas();
exitCommand = new Command("Exit", Command.EXIT, 1);
d.addCommand(exitCommand);
d.setCommandListener(this);
display.setCurrent(d);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command c, Displayable s) {
notifyDestroyed();
}
}
class RectCanvas extends Canvas {
int width = 0;
int height = 0;
public void paint(Graphics g) {
width = getWidth();
height = getHeight();
g.setGrayScale(255);
g.fillRect(0, 0, width - 1, height - 1);
g.setGrayScale(0);
g.drawRect(0, 0, width - 1, height - 1);
g.drawRect(5, 5, 40, 40);
g.fillRoundRect(25, 25, 40, 40, 15, 15);
}
}