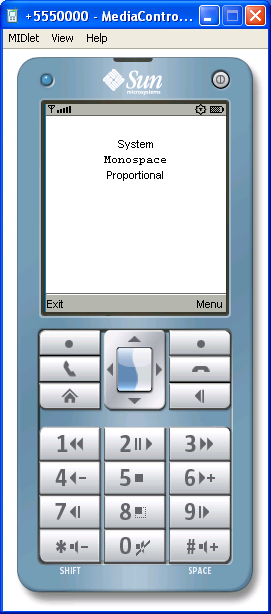
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Font;
import javax.microedition.lcdui.Graphics;
import javax.microedition.midlet.MIDlet;
public class FontMIDlet extends MIDlet implements CommandListener {
private FontCanvas mFontCanvas;
private Command mBoldCommand, mItalicCommand, mUnderlineCommand;
public FontMIDlet() {
mFontCanvas = new FontCanvas();
mBoldCommand = new Command("Bold", Command.SCREEN, 0);
mItalicCommand = new Command("Italic", Command.SCREEN, 0);
mUnderlineCommand = new Command("Underline", Command.SCREEN, 0);
Command exitCommand = new Command("Exit", Command.EXIT, 0);
mFontCanvas.addCommand(mBoldCommand);
mFontCanvas.addCommand(mItalicCommand);
mFontCanvas.addCommand(mUnderlineCommand);
mFontCanvas.addCommand(exitCommand);
mFontCanvas.setCommandListener(this);
}
public void startApp() {
Display.getDisplay(this).setCurrent(mFontCanvas);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command c, Displayable s) {
if (c.getCommandType() == Command.EXIT) {
notifyDestroyed();
return;
}
boolean isBold = mFontCanvas.isBold() ^ (c == mBoldCommand);
boolean isItalic = mFontCanvas.isItalic() ^ (c == mItalicCommand);
boolean isUnderline = mFontCanvas.isUnderline() ^ (c == mUnderlineCommand);
int style = (isBold ? Font.STYLE_BOLD : 0) | (isItalic ? Font.STYLE_ITALIC : 0)
| (isUnderline ? Font.STYLE_UNDERLINED : 0);
mFontCanvas.setStyle(style);
mFontCanvas.repaint();
}
}
class FontCanvas extends Canvas {
private Font mSystemFont, mMonospaceFont, mProportionalFont;
public FontCanvas() {
this(Font.STYLE_PLAIN);
}
public FontCanvas(int style) {
setStyle(style);
}
public void setStyle(int style) {
mSystemFont = Font.getFont(Font.FACE_SYSTEM, style, Font.SIZE_MEDIUM);
mMonospaceFont = Font.getFont(Font.FACE_MONOSPACE, style, Font.SIZE_MEDIUM);
mProportionalFont = Font.getFont(Font.FACE_PROPORTIONAL, style, Font.SIZE_MEDIUM);
}
public boolean isBold() {
return mSystemFont.isBold();
}
public boolean isItalic() {
return mSystemFont.isItalic();
}
public boolean isUnderline() {
return mSystemFont.isUnderlined();
}
public void paint(Graphics g) {
int w = getWidth();
int h = getHeight();
int x = w / 2;
int y = 20;
y += showFont(g, "System", x, y, mSystemFont);
y += showFont(g, "Monospace", x, y, mMonospaceFont);
y += showFont(g, "Proportional", x, y, mProportionalFont);
}
private int showFont(Graphics g, String s, int x, int y, Font f) {
g.setFont(f);
g.drawString(s, x, y, Graphics.TOP | Graphics.HCENTER);
return f.getHeight();
}
}