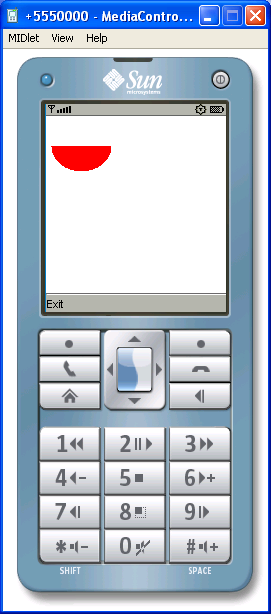
import javax.microedition.lcdui.Alert;
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Graphics;
import javax.microedition.lcdui.Image;
import javax.microedition.midlet.MIDlet;
public class J2MEMutableImageExample extends MIDlet {
private Display display;
private MyCanvas canvas;
public J2MEMutableImageExample() {
display = Display.getDisplay(this);
canvas = new MyCanvas(this);
}
protected void startApp() {
display.setCurrent(canvas);
}
protected void pauseApp() {
}
protected void destroyApp(boolean unconditional) {
}
public void exitMIDlet() {
destroyApp(true);
notifyDestroyed();
}
class MyCanvas extends Canvas implements CommandListener {
private Command exit;
private J2MEMutableImageExample mutableImageExample;
private Image image = null;
public MyCanvas(J2MEMutableImageExample mutableImageExample) {
this.mutableImageExample = mutableImageExample;
exit = new Command("Exit", Command.EXIT, 1);
addCommand(exit);
setCommandListener(this);
try {
image = Image.createImage(70, 70);
Graphics graphics = image.getGraphics();
graphics.setColor(255, 0, 0);
graphics.fillArc(10, 10, 60, 50, 180, 180);
} catch (Exception error) {
Alert alert = new Alert("Failure", "Creating Image", null, null);
alert.setTimeout(Alert.FOREVER);
display.setCurrent(alert);
}
}
protected void paint(Graphics graphics) {
if (image != null) {
graphics.setColor(255, 255, 255);
graphics.fillRect(0, 0, getWidth(), getHeight());
graphics.drawImage(image, 30, 30, Graphics.VCENTER | Graphics.HCENTER);
}
}
public void commandAction(Command command, Displayable display) {
if (command == exit) {
mutableImageExample.exitMIDlet();
}
}
}
}