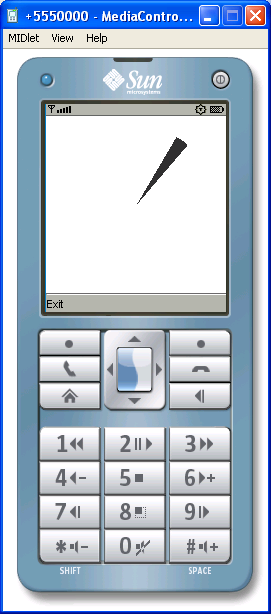
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Graphics;
import javax.microedition.midlet.MIDlet;
public class J2MESweep extends MIDlet {
public void startApp() {
final SweepCanvas sweeper = new SweepCanvas();
sweeper.start();
sweeper.addCommand(new Command("Exit", Command.EXIT, 0));
sweeper.setCommandListener(new CommandListener() {
public void commandAction(Command c, Displayable s) {
sweeper.stop();
notifyDestroyed();
}
});
Display.getDisplay(this).setCurrent(sweeper);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
class SweepCanvas extends Canvas implements Runnable {
private boolean mTrucking;
private int mTheta = 0;
private int mBorder = 10;
private int mDelay = 50;
public void start() {
mTrucking = true;
Thread t = new Thread(this);
t.start();
}
public void stop() {
mTrucking = false;
}
public void paint(Graphics g) {
int width = getWidth();
int height = getHeight();
g.setGrayScale(255);
g.fillRect(0, 0, width - 1, height - 1);
int x = mBorder;
int y = mBorder;
int w = width - mBorder * 2;
int h = height - mBorder * 2;
for (int i = 0; i < 8; i++) {
g.setGrayScale((8 - i) * 32 - 16);
g.fillArc(x, y, w, h, mTheta + i * 10, 10);
}
}
public void run() {
while (mTrucking) {
mTheta = (mTheta + 1) % 360;
repaint();
try {
Thread.sleep(mDelay);
} catch (InterruptedException ie) {
}
}
}
}