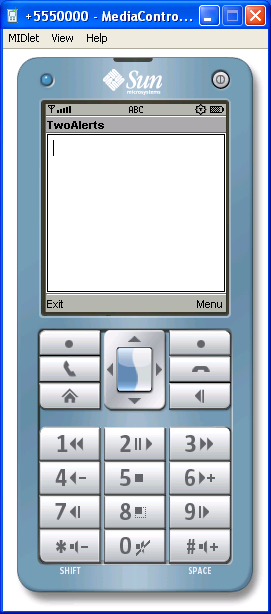
import javax.microedition.lcdui.Alert;
import javax.microedition.lcdui.AlertType;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.TextBox;
import javax.microedition.lcdui.TextField;
import javax.microedition.midlet.MIDlet;
public class J2METwoAlerts extends MIDlet implements CommandListener {
private Display mDisplay;
private TextBox mTextBox= new TextBox("TwoAlerts", "", 32, TextField.ANY);
private Alert mTimedAlert = new Alert("Network error", "error occurred", null, AlertType.INFO);
private Alert mModalAlert= new Alert("About TwoAlerts","Alerts.", null, AlertType.INFO);
private Command mAboutCommand, mGoCommand, mExitCommand;
public J2METwoAlerts() {
mAboutCommand = new Command("About", Command.SCREEN, 1);
mGoCommand = new Command("Go", Command.SCREEN, 1);
mExitCommand = new Command("Exit", Command.EXIT, 2);
mTextBox.addCommand(mAboutCommand);
mTextBox.addCommand(mGoCommand);
mTextBox.addCommand(mExitCommand);
mTextBox.setCommandListener(this);
mModalAlert.setTimeout(Alert.FOREVER);
}
public void startApp() {
mDisplay = Display.getDisplay(this);
mDisplay.setCurrent(mTextBox);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
public void commandAction(Command c, Displayable s) {
if (c == mAboutCommand)
mDisplay.setCurrent(mModalAlert);
else if (c == mGoCommand)
mDisplay.setCurrent(mTimedAlert, mTextBox);
else if (c == mExitCommand)
notifyDestroyed();
}
}