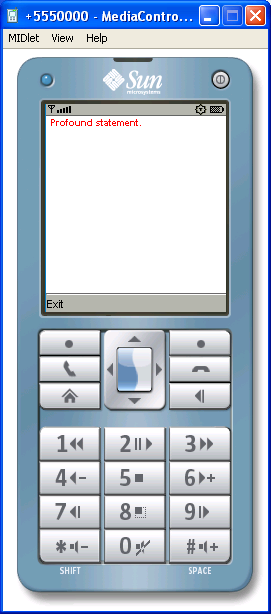
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Font;
import javax.microedition.lcdui.Graphics;
import javax.microedition.midlet.MIDlet;
public class J2METextExample extends MIDlet {
private Display display;
private MyCanvas canvas;
public J2METextExample() {
display = Display.getDisplay(this);
canvas = new MyCanvas(this);
}
protected void startApp() {
display.setCurrent(canvas);
}
protected void pauseApp() {
}
protected void destroyApp(boolean unconditional) {
}
public void exitMIDlet() {
destroyApp(true);
notifyDestroyed();
}
}
class MyCanvas extends Canvas implements CommandListener {
private Command exit;
private J2METextExample textExample;
public MyCanvas(J2METextExample textExample) {
this.textExample = textExample;
exit = new Command("Exit", Command.EXIT, 1);
addCommand(exit);
setCommandListener(this);
}
protected void paint(Graphics graphics) {
graphics.setColor(255, 0, 0);
graphics.setFont(Font.getFont(Font.FACE_PROPORTIONAL, Font.STYLE_BOLD, Font.SIZE_SMALL));
graphics.drawString("Profound statement.", 50, 10, Graphics.HCENTER | Graphics.BASELINE);
}
public void commandAction(Command command, Displayable displayable) {
if (command == exit) {
textExample.exitMIDlet();
}
}
}