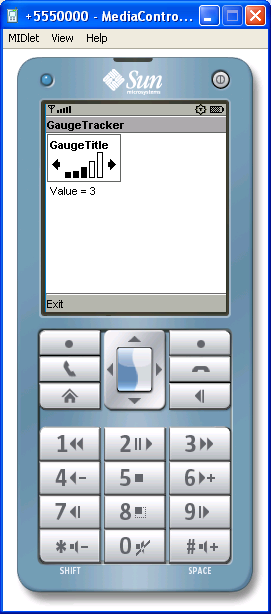
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Form;
import javax.microedition.lcdui.Gauge;
import javax.microedition.lcdui.Item;
import javax.microedition.lcdui.ItemStateListener;
import javax.microedition.lcdui.StringItem;
import javax.microedition.midlet.MIDlet;
public class J2MEGaugeTracker extends MIDlet implements ItemStateListener {
private Gauge mGauge;
private StringItem mStringItem;
public J2MEGaugeTracker() {
int initialValue = 5;
mGauge = new Gauge("GaugeTitle", true, 10, initialValue);
mStringItem = new StringItem(null, "[value]");
itemStateChanged(mGauge);
}
public void itemStateChanged(Item item) {
if (item == mGauge)
mStringItem.setText("Value = " + mGauge.getValue());
}
public void startApp() {
Form form = new Form("GaugeTracker");
form.append(mGauge);
form.append(mStringItem);
form.setItemStateListener(this);
Display.getDisplay(this).setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}