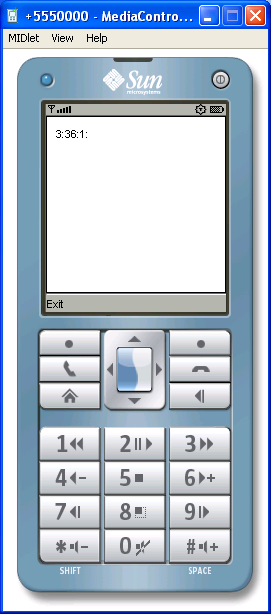
import java.util.Calendar;
import java.util.Date;
import java.util.Timer;
import java.util.TimerTask;
import javax.microedition.lcdui.Canvas;
import javax.microedition.lcdui.Command;
import javax.microedition.lcdui.CommandListener;
import javax.microedition.lcdui.Display;
import javax.microedition.lcdui.Displayable;
import javax.microedition.lcdui.Graphics;
import javax.microedition.midlet.MIDlet;
public class J2MEFixedRateSchedule extends MIDlet {
private Timer aTimer;
private ClockTimerTask aTimerTask;
Displayable d = new ClockCanvas();
private Date currentTime= new Date();
private Calendar now = Calendar.getInstance();
private String nowString = "";
public void startApp() {
d.addCommand(new Command("Exit", Command.EXIT, 0));
d.setCommandListener(new CommandListener() {
public void commandAction(Command c, Displayable s) {
notifyDestroyed();
}
});
now.setTime(currentTime);
nowString = now.get(Calendar.HOUR) + ":" + now.get(Calendar.MINUTE) + ":"
+ now.get(Calendar.SECOND) + ":";
aTimer = new Timer();
aTimerTask = new ClockTimerTask();
aTimer.scheduleAtFixedRate(aTimerTask, 10, 1000);
Display.getDisplay(this).setCurrent(d);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
class ClockCanvas extends Canvas {
public void paint(Graphics g) {
int width = getWidth();
int height = getHeight();
g.setGrayScale(255);
g.fillRect(0, 0, width - 1, height - 1);
g.setGrayScale(0);
g.drawRect(0, 0, width - 1, height - 1);
g.drawString(nowString, 10, 10, Graphics.TOP | Graphics.LEFT);
}
}
class ClockTimerTask extends TimerTask {
public final void run() {
currentTime = new Date();
now = Calendar.getInstance();
now.setTime(currentTime);
nowString = now.get(Calendar.HOUR) + ":" + now.get(Calendar.MINUTE) + ":"
+ now.get(Calendar.SECOND) + ":";
((ClockCanvas) d).repaint();
}
}
}