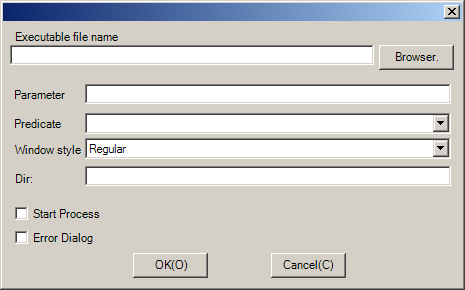
Imports System.Diagnostics
Imports System.Windows.Forms
public class ProcessStartForm
public Shared Sub Main
Application.Run(New FormStartInfo)
End Sub
End class
Public Class FormStartInfo
Private Sub ListVerb(ByVal filename As String)
cbxVerb.Items.Clear()
Dim sinfo As ProcessStartInfo = New ProcessStartInfo(filename)
cbxVerb.Items.AddRange(sinfo.Verbs)
End Sub
Private Sub FormStartInfo_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
cbxStyle.SelectedIndex = 2
End Sub
Private Sub txtFileName_Leave(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles txtFileName.Leave
ListVerb(txtFileName.Text)
End Sub
Private Sub btnExplorer_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnExplorer.Click
Dim dlg As OpenFileDialog = New OpenFileDialog()
dlg.CheckFileExists = True
dlg.Filter = "(*.exe)|*.exe|(*.*)|*.*"
If dlg.ShowDialog = System.Windows.Forms.DialogResult.OK Then
txtFileName.Text = dlg.FileName
ListVerb(dlg.FileName)
End If
End Sub
Private Sub btnOK_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnOK.Click
Dim si As ProcessStartInfo = New ProcessStartInfo()
si.FileName = txtFileName.Text
si.Arguments = txtParam.Text
si.ErrorDialog = chkErrDlg.Checked
si.UseShellExecute = chkUseShell.Checked
If cbxVerb.SelectedIndex <> -1 Then si.Verb = cbxVerb.Text
si.WorkingDirectory = txtWorkDir.Text
If cbxStyle.SelectedIndex = 0 Then
si.WindowStyle = ProcessWindowStyle.Maximized
ElseIf cbxStyle.SelectedIndex = 1 Then
si.WindowStyle = ProcessWindowStyle.Minimized
Else
si.WindowStyle = ProcessWindowStyle.Normal
End If
Try
Process.Start(si)
Catch ex As Exception
Console.WriteLine(ex.Message)
End Try
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class FormStartInfo
Inherits System.Windows.Forms.Form
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
Private components As System.ComponentModel.IContainer
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.cbxStyle = New System.Windows.Forms.ComboBox
Me.cbxVerb = New System.Windows.Forms.ComboBox
Me.txtWorkDir = New System.Windows.Forms.TextBox
Me.txtParam = New System.Windows.Forms.TextBox
Me.btnCancel = New System.Windows.Forms.Button
Me.btnOK = New System.Windows.Forms.Button
Me.chkErrDlg = New System.Windows.Forms.CheckBox
Me.chkUseShell = New System.Windows.Forms.CheckBox
Me.label5 = New System.Windows.Forms.Label
Me.label4 = New System.Windows.Forms.Label
Me.label3 = New System.Windows.Forms.Label
Me.label2 = New System.Windows.Forms.Label
Me.btnExplorer = New System.Windows.Forms.Button
Me.txtFileName = New System.Windows.Forms.TextBox
Me.label1 = New System.Windows.Forms.Label
Me.SuspendLayout()
'
'cbxStyle
'
Me.cbxStyle.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList
Me.cbxStyle.FormattingEnabled = True
Me.cbxStyle.Items.AddRange(New Object() {"Maximized", "Minimized", "Regular"})
Me.cbxStyle.Location = New System.Drawing.Point(109, 134)
Me.cbxStyle.Margin = New System.Windows.Forms.Padding(4)
Me.cbxStyle.Name = "cbxStyle"
Me.cbxStyle.Size = New System.Drawing.Size(487, 23)
Me.cbxStyle.TabIndex = 29
'
'cbxVerb
'
Me.cbxVerb.DropDownStyle = System.Windows.Forms.ComboBoxStyle.DropDownList
Me.cbxVerb.FormattingEnabled = True
Me.cbxVerb.Location = New System.Drawing.Point(109, 105)
Me.cbxVerb.Margin = New System.Windows.Forms.Padding(4)
Me.cbxVerb.Name = "cbxVerb"
Me.cbxVerb.Size = New System.Drawing.Size(487, 23)
Me.cbxVerb.TabIndex = 28
'
'txtWorkDir
'
Me.txtWorkDir.Location = New System.Drawing.Point(109, 166)
Me.txtWorkDir.Margin = New System.Windows.Forms.Padding(4)
Me.txtWorkDir.Name = "txtWorkDir"
Me.txtWorkDir.Size = New System.Drawing.Size(487, 25)
Me.txtWorkDir.TabIndex = 27
'
'txtParam
'
Me.txtParam.Location = New System.Drawing.Point(109, 71)
Me.txtParam.Margin = New System.Windows.Forms.Padding(4)
Me.txtParam.Name = "txtParam"
Me.txtParam.Size = New System.Drawing.Size(487, 25)
Me.txtParam.TabIndex = 26
'
'btnCancel
'
Me.btnCancel.DialogResult = System.Windows.Forms.DialogResult.Cancel
Me.btnCancel.Location = New System.Drawing.Point(358, 266)
Me.btnCancel.Margin = New System.Windows.Forms.Padding(4)
Me.btnCancel.Name = "btnCancel"
Me.btnCancel.Size = New System.Drawing.Size(100, 29)
Me.btnCancel.TabIndex = 25
Me.btnCancel.Text = "Cancel(&C)"
Me.btnCancel.UseVisualStyleBackColor = True
'
'btnOK
'
Me.btnOK.DialogResult = System.Windows.Forms.DialogResult.OK
Me.btnOK.Location = New System.Drawing.Point(174, 266)
Me.btnOK.Margin = New System.Windows.Forms.Padding(4)
Me.btnOK.Name = "btnOK"
Me.btnOK.Size = New System.Drawing.Size(100, 29)
Me.btnOK.TabIndex = 24
Me.btnOK.Text = "OK(&O)"
Me.btnOK.UseVisualStyleBackColor = True
'
'chkErrDlg
'
Me.chkErrDlg.AutoSize = True
Me.chkErrDlg.Location = New System.Drawing.Point(16, 239)
Me.chkErrDlg.Margin = New System.Windows.Forms.Padding(4)
Me.chkErrDlg.Name = "chkErrDlg"
Me.chkErrDlg.Size = New System.Drawing.Size(134, 19)
Me.chkErrDlg.TabIndex = 23
Me.chkErrDlg.Text = "Error Dialog"
Me.chkErrDlg.UseVisualStyleBackColor = True
'
'chkUseShell
'
Me.chkUseShell.AutoSize = True
Me.chkUseShell.Location = New System.Drawing.Point(16, 211)
Me.chkUseShell.Margin = New System.Windows.Forms.Padding(4)
Me.chkUseShell.Name = "chkUseShell"
Me.chkUseShell.Size = New System.Drawing.Size(209, 19)
Me.chkUseShell.TabIndex = 22
Me.chkUseShell.Text = "Start Process"
Me.chkUseShell.UseVisualStyleBackColor = True
'
'label5
'
Me.label5.AutoSize = True
Me.label5.Location = New System.Drawing.Point(13, 173)
Me.label5.Margin = New System.Windows.Forms.Padding(4, 0, 4, 0)
Me.label5.Name = "label5"
Me.label5.Size = New System.Drawing.Size(82, 15)
Me.label5.TabIndex = 21
Me.label5.Text = "Dir:"
'
'label4
'
Me.label4.AutoSize = True
Me.label4.Location = New System.Drawing.Point(13, 140)
Me.label4.Margin = New System.Windows.Forms.Padding(4, 0, 4, 0)
Me.label4.Name = "label4"
Me.label4.Size = New System.Drawing.Size(82, 15)
Me.label4.TabIndex = 20
Me.label4.Text = "Window style"
'
'label3
'
Me.label3.AutoSize = True
Me.label3.Location = New System.Drawing.Point(12, 110)
Me.label3.Margin = New System.Windows.Forms.Padding(4, 0, 4, 0)
Me.label3.Name = "label3"
Me.label3.Size = New System.Drawing.Size(52, 15)
Me.label3.TabIndex = 19
Me.label3.Text = "Predicate"
'
'label2
'
Me.label2.AutoSize = True
Me.label2.Location = New System.Drawing.Point(12, 76)
Me.label2.Margin = New System.Windows.Forms.Padding(4, 0, 4, 0)
Me.label2.Name = "label2"
Me.label2.Size = New System.Drawing.Size(52, 15)
Me.label2.TabIndex = 18
Me.label2.Text = "Parameter"
'
'btnExplorer
'
Me.btnExplorer.Location = New System.Drawing.Point(502, 27)
Me.btnExplorer.Margin = New System.Windows.Forms.Padding(4)
Me.btnExplorer.Name = "btnExplorer"
Me.btnExplorer.Size = New System.Drawing.Size(100, 29)
Me.btnExplorer.TabIndex = 17
Me.btnExplorer.Text = "Browser."
Me.btnExplorer.UseVisualStyleBackColor = True
'
'txtFileName
'
Me.txtFileName.Location = New System.Drawing.Point(9, 27)
Me.txtFileName.Margin = New System.Windows.Forms.Padding(4)
Me.txtFileName.Name = "txtFileName"
Me.txtFileName.Size = New System.Drawing.Size(484, 25)
Me.txtFileName.TabIndex = 16
'
'label1
'
Me.label1.AutoSize = True
Me.label1.Location = New System.Drawing.Point(13, 9)
Me.label1.Margin = New System.Windows.Forms.Padding(4, 0, 4, 0)
Me.label1.Name = "label1"
Me.label1.Size = New System.Drawing.Size(307, 15)
Me.label1.TabIndex = 15
Me.label1.Text = "Executable file name"
'
'FormStartInfo
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(8.0!, 15.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(612, 306)
Me.Controls.Add(Me.cbxStyle)
Me.Controls.Add(Me.cbxVerb)
Me.Controls.Add(Me.txtWorkDir)
Me.Controls.Add(Me.txtParam)
Me.Controls.Add(Me.btnCancel)
Me.Controls.Add(Me.btnOK)
Me.Controls.Add(Me.chkErrDlg)
Me.Controls.Add(Me.chkUseShell)
Me.Controls.Add(Me.label5)
Me.Controls.Add(Me.label4)
Me.Controls.Add(Me.label3)
Me.Controls.Add(Me.label2)
Me.Controls.Add(Me.btnExplorer)
Me.Controls.Add(Me.txtFileName)
Me.Controls.Add(Me.label1)
Me.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog
Me.MaximizeBox = False
Me.MinimizeBox = False
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Private WithEvents cbxStyle As System.Windows.Forms.ComboBox
Private WithEvents cbxVerb As System.Windows.Forms.ComboBox
Private WithEvents txtWorkDir As System.Windows.Forms.TextBox
Private WithEvents txtParam As System.Windows.Forms.TextBox
Private WithEvents btnCancel As System.Windows.Forms.Button
Private WithEvents btnOK As System.Windows.Forms.Button
Private WithEvents chkErrDlg As System.Windows.Forms.CheckBox
Private WithEvents chkUseShell As System.Windows.Forms.CheckBox
Private WithEvents label5 As System.Windows.Forms.Label
Private WithEvents label4 As System.Windows.Forms.Label
Private WithEvents label3 As System.Windows.Forms.Label
Private WithEvents label2 As System.Windows.Forms.Label
Private WithEvents btnExplorer As System.Windows.Forms.Button
Private WithEvents txtFileName As System.Windows.Forms.TextBox
Private WithEvents label1 As System.Windows.Forms.Label
End Class