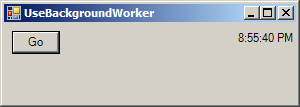
Imports System.Windows.Forms
public class BackgroundWorker
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
' Display the current time.
Private Sub tmrUpdateClock_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles tmrUpdateClock.Tick
lblTime.Text = Now.ToString("T")
End Sub
' Start the asynchronous calculation.
Private Sub btnGo_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnGo.Click
btnGo.Enabled = False
lblWorking.Text = "Working..."
Me.Cursor = Cursors.WaitCursor
Application.DoEvents()
bgwLongCalculation.RunWorkerAsync(5)
End Sub
Private Sub bgwLongCalculation_DoWork(ByVal sender As Object, ByVal e As System.ComponentModel.DoWorkEventArgs) Handles bgwLongCalculation.DoWork
For i As Integer = 0 To 100000
For j As Integer = 0 To 100000
For k As Integer = 0 To 100000
Next k
Next j
Next i
End Sub
' The long task is done.
Private Sub bgwLongCalculation_RunWorkerCompleted(ByVal sender As Object, ByVal e As System.ComponentModel.RunWorkerCompletedEventArgs) Handles bgwLongCalculation.RunWorkerCompleted
btnGo.Enabled = True
lblWorking.Text = "Done..."
Me.Cursor = Cursors.Default
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Public Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.components = New System.ComponentModel.Container
Me.btnGo = New System.Windows.Forms.Button
Me.lblTime = New System.Windows.Forms.Label
Me.tmrUpdateClock = New System.Windows.Forms.Timer(Me.components)
Me.bgwLongCalculation = New System.ComponentModel.BackgroundWorker
Me.lblWorking = New System.Windows.Forms.Label
Me.SuspendLayout()
'
'btnGo
'
Me.btnGo.Location = New System.Drawing.Point(8, 8)
Me.btnGo.Name = "btnGo"
Me.btnGo.Size = New System.Drawing.Size(48, 23)
Me.btnGo.TabIndex = 0
Me.btnGo.Text = "Go"
'
'lblTime
'
Me.lblTime.AutoSize = True
Me.lblTime.Location = New System.Drawing.Point(232, 8)
Me.lblTime.Name = "lblTime"
Me.lblTime.Size = New System.Drawing.Size(36, 13)
Me.lblTime.TabIndex = 1
Me.lblTime.Text = "lblTime"
'
'tmrUpdateClock
'
Me.tmrUpdateClock.Enabled = True
Me.tmrUpdateClock.Interval = 250
'
'bgwLongCalculation
'
'
'lblWorking
'
Me.lblWorking.Location = New System.Drawing.Point(80, 8)
Me.lblWorking.Name = "lblWorking"
Me.lblWorking.Size = New System.Drawing.Size(112, 24)
Me.lblWorking.TabIndex = 2
Me.lblWorking.TextAlign = System.Drawing.ContentAlignment.MiddleLeft
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 13.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(292, 80)
Me.Controls.Add(Me.lblWorking)
Me.Controls.Add(Me.lblTime)
Me.Controls.Add(Me.btnGo)
Me.Name = "Form1"
Me.Text = "UseBackgroundWorker"
Me.ResumeLayout(False)
Me.PerformLayout()
End Sub
Friend WithEvents btnGo As System.Windows.Forms.Button
Friend WithEvents lblTime As System.Windows.Forms.Label
Friend WithEvents tmrUpdateClock As System.Windows.Forms.Timer
Friend WithEvents bgwLongCalculation As System.ComponentModel.BackgroundWorker
Friend WithEvents lblWorking As System.Windows.Forms.Label
End Class