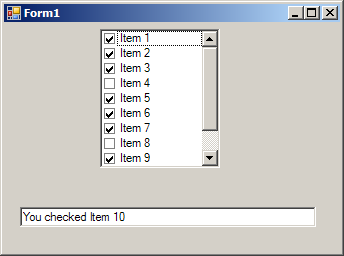
Imports System.Drawing
Imports System.Drawing.Drawing2D
Imports System.Windows.Forms
public class CheckBoxListAddValueSelectionEvent
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents CheckedListBox1 As System.Windows.Forms.CheckedListBox
Friend WithEvents TextBox1 As System.Windows.Forms.TextBox
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.CheckedListBox1 = New System.Windows.Forms.CheckedListBox
Me.TextBox1 = New System.Windows.Forms.TextBox
Me.SuspendLayout()
'
'CheckedListBox1
'
Me.CheckedListBox1.CheckOnClick = True
Me.CheckedListBox1.Font = New System.Drawing.Font("Microsoft Sans Serif", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.CheckedListBox1.Location = New System.Drawing.Point(96, 6)
Me.CheckedListBox1.Name = "CheckedListBox1"
Me.CheckedListBox1.Size = New System.Drawing.Size(120, 150)
Me.CheckedListBox1.TabIndex = 0
'
'TextBox1
'
Me.TextBox1.Font = New System.Drawing.Font("Microsoft Sans Serif", 8.25!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.TextBox1.Location = New System.Drawing.Point(16, 184)
Me.TextBox1.Name = "TextBox1"
Me.TextBox1.Size = New System.Drawing.Size(296, 20)
Me.TextBox1.TabIndex = 2
Me.TextBox1.Text = "TextBox1"
'
'Form1
'
Me.AutoScaleBaseSize = New System.Drawing.Size(15, 37)
Me.ClientSize = New System.Drawing.Size(336, 229)
Me.Controls.Add(Me.TextBox1)
Me.Controls.Add(Me.CheckedListBox1)
Me.Font = New System.Drawing.Font("Microsoft Sans Serif", 24.0!, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.Name = "Form1"
Me.Text = "Form1"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
CheckedListBox1.Items.Add("Item 1", True)
CheckedListBox1.Items.Add("Item 2", True)
CheckedListBox1.Items.Add("Item 3", True)
CheckedListBox1.Items.Add("Item 4", False)
CheckedListBox1.Items.Add("Item 5", True)
CheckedListBox1.Items.Add("Item 6", True)
CheckedListBox1.Items.Add("Item 7", True)
CheckedListBox1.Items.Add("Item 8", False)
CheckedListBox1.Items.Add("Item 9", True)
CheckedListBox1.Items.Add("Item 10", True)
CheckedListBox1.Items.Add("Item 11", True)
End Sub
Private Sub CheckedListBox1_ItemCheck(ByVal sender As Object, ByVal e As System.Windows.Forms.ItemCheckEventArgs) Handles CheckedListBox1.ItemCheck
Select Case e.NewValue
Case CheckState.Checked
TextBox1.Text = "You checked Item " & e.Index
Case CheckState.Unchecked
TextBox1.Text = "You unchecked Item " & e.Index
End Select
End Sub
End Class