Form Inheritance : Form « GUI « VB.Net Tutorial
- VB.Net Tutorial
- GUI
- Form
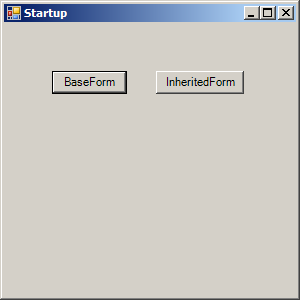
Imports System.Windows.Forms
public class FormInheritance
public Shared Sub Main
Application.Run(New Startup)
End Sub
End class
Public Class Startup
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Friend WithEvents Button1 As System.Windows.Forms.Button
Friend WithEvents Button2 As System.Windows.Forms.Button
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.Button1 = New System.Windows.Forms.Button()
Me.Button2 = New System.Windows.Forms.Button()
Me.SuspendLayout()
'
'Button1
'
Me.Button1.Location = New System.Drawing.Point(48, 48)
Me.Button1.Name = "Button1"
Me.Button1.TabIndex = 0
Me.Button1.Text = "BaseForm"
'
'Button2
'
Me.Button2.Location = New System.Drawing.Point(152, 48)
Me.Button2.Name = "Button2"
Me.Button2.Size = New System.Drawing.Size(88, 23)
Me.Button2.TabIndex = 1
Me.Button2.Text = "InheritedForm"
'
'Startup
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.Button2, Me.Button1})
Me.Name = "Startup"
Me.Text = "Startup"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
dim frm1 as new BaseForm()
frm1.Show()
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
dim frm2 as new InheritedForm()
frm2.Show()
End Sub
End Class
Public Class BaseForm
Inherits System.Windows.Forms.Form
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
Protected WithEvents lblHeading As System.Windows.Forms.Label
Protected WithEvents btn As System.Windows.Forms.Button
Protected WithEvents lblOutput As System.Windows.Forms.Label
Private WithEvents Label3 As System.Windows.Forms.Label
Private WithEvents Label2 As System.Windows.Forms.Label
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.lblHeading = New System.Windows.Forms.Label()
Me.btn = New System.Windows.Forms.Button()
Me.lblOutput = New System.Windows.Forms.Label()
Me.Label3 = New System.Windows.Forms.Label()
Me.Label2 = New System.Windows.Forms.Label()
Me.SuspendLayout()
'
'lblHeading
'
Me.lblHeading.Font = New System.Drawing.Font("Microsoft Sans Serif", 15.75!, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, CType(0, Byte))
Me.lblHeading.Location = New System.Drawing.Point(24, 16)
Me.lblHeading.Name = "lblHeading"
Me.lblHeading.Size = New System.Drawing.Size(248, 23)
Me.lblHeading.TabIndex = 0
Me.lblHeading.Text = "Base Form"
Me.lblHeading.TextAlign = System.Drawing.ContentAlignment.MiddleCenter
'
'btn
'
Me.btn.Location = New System.Drawing.Point(96, 72)
Me.btn.Name = "btn"
Me.btn.TabIndex = 1
Me.btn.Text = "Time"
'
'lblOutput
'
Me.lblOutput.Location = New System.Drawing.Point(64, 128)
Me.lblOutput.Name = "lblOutput"
Me.lblOutput.Size = New System.Drawing.Size(136, 23)
Me.lblOutput.TabIndex = 2
Me.lblOutput.TextAlign = System.Drawing.ContentAlignment.MiddleCenter
'
'Label3
'
Me.Label3.Location = New System.Drawing.Point(80, 200)
Me.Label3.Name = "Label3"
Me.Label3.TabIndex = 3
Me.Label3.Text = "Base Form"
Me.Label3.TextAlign = System.Drawing.ContentAlignment.MiddleCenter
'
'Label2
'
Me.Label2.Location = New System.Drawing.Point(40, 232)
Me.Label2.Name = "Label2"
Me.Label2.Size = New System.Drawing.Size(216, 23)
Me.Label2.TabIndex = 4
Me.Label2.Text = "Created in Visual Studio .NET"
Me.Label2.TextAlign = System.Drawing.ContentAlignment.MiddleCenter
'
'BaseForm
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.Label2, Me.Label3, Me.lblOutput, Me.btn, Me.lblHeading})
Me.Name = "BaseForm"
Me.Text = "Visual Form Inheritance"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btn_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn.Click
lblOutput.Text = "The time is: " + DateTime.Now.ToString("T")
End Sub
End Class
Public Class InheritedForm
Inherits BaseForm
#Region " Windows Form Designer generated code "
Public Sub New()
MyBase.New()
'This call is required by the Windows Form Designer.
InitializeComponent()
'Add any initialization after the InitializeComponent() call
btn.Text = "Date"
lblHeading.Text = "Inherited Form"
End Sub
'Form overrides dispose to clean up the component list.
Protected Overloads Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing Then
If Not (components Is Nothing) Then
components.Dispose()
End If
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> Private Sub InitializeComponent()
Me.SuspendLayout()
'
'lblHeading
'
Me.lblHeading.BackColor = System.Drawing.Color.Yellow
Me.lblHeading.Visible = True
'
'btn
'
Me.btn.BackColor = System.Drawing.Color.Yellow
Me.btn.Visible = True
'
'lblOutput
'
Me.lblOutput.BackColor = System.Drawing.Color.Yellow
Me.lblOutput.Visible = True
'
'InheritedForm
'
Me.AutoScaleBaseSize = New System.Drawing.Size(5, 13)
Me.BackColor = System.Drawing.Color.Yellow
Me.ClientSize = New System.Drawing.Size(292, 273)
Me.Controls.AddRange(New System.Windows.Forms.Control() {Me.lblOutput, Me.btn, Me.lblHeading})
Me.Name = "InheritedForm"
Me.ResumeLayout(False)
End Sub
#End Region
Private Sub btn_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btn.Click
lblOutput.Text = "Today is: " + DateTime.Now.ToString("D")
End Sub
End Class