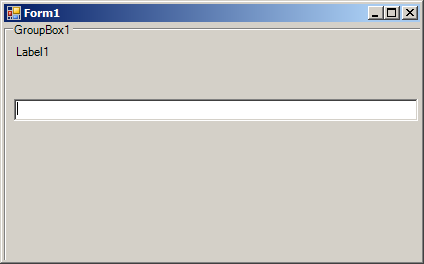
Imports System.Windows.Forms
public class FormResizeEvent
public Shared Sub Main
Application.Run(New Form1)
End Sub
End class
Public Class Form1
Private Sub Form1_Resize(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Resize
GroupBox1.Top = 0
GroupBox1.Left = 0
GroupBox1.Width = Me.Width
GroupBox1.Height = Me.Height
Label1.Left = GroupBox1.Top + 10
Label1.Top = GroupBox1.Left + 20
TextBox1.Top = Label1.Top + 50
TextBox1.Left = Label1.Left
TextBox1.Width = GroupBox1.Width - 20
End Sub
End Class
<Global.Microsoft.VisualBasic.CompilerServices.DesignerGenerated()> _
Partial Class Form1
Inherits System.Windows.Forms.Form
'Form overrides dispose to clean up the component list.
<System.Diagnostics.DebuggerNonUserCode()> _
Protected Overrides Sub Dispose(ByVal disposing As Boolean)
If disposing AndAlso components IsNot Nothing Then
components.Dispose()
End If
MyBase.Dispose(disposing)
End Sub
'Required by the Windows Form Designer
Private components As System.ComponentModel.IContainer
'NOTE: The following procedure is required by the Windows Form Designer
'It can be modified using the Windows Form Designer.
'Do not modify it using the code editor.
<System.Diagnostics.DebuggerStepThrough()> _
Private Sub InitializeComponent()
Me.GroupBox1 = New System.Windows.Forms.GroupBox
Me.TextBox1 = New System.Windows.Forms.TextBox
Me.Label1 = New System.Windows.Forms.Label
Me.GroupBox1.SuspendLayout()
Me.SuspendLayout()
'
'GroupBox1
'
Me.GroupBox1.Controls.Add(Me.TextBox1)
Me.GroupBox1.Controls.Add(Me.Label1)
Me.GroupBox1.Location = New System.Drawing.Point(12, 12)
Me.GroupBox1.Name = "GroupBox1"
Me.GroupBox1.Size = New System.Drawing.Size(392, 195)
Me.GroupBox1.TabIndex = 0
Me.GroupBox1.TabStop = False
Me.GroupBox1.Text = "GroupBox1"
'
'TextBox1
'
Me.TextBox1.Location = New System.Drawing.Point(32, 62)
Me.TextBox1.Multiline = True
Me.TextBox1.Name = "TextBox1"
Me.TextBox1.Size = New System.Drawing.Size(331, 21)
Me.TextBox1.TabIndex = 5
'
'Label1
'
Me.Label1.AutoSize = True
Me.Label1.Location = New System.Drawing.Point(30, 30)
Me.Label1.Name = "Label1"
Me.Label1.Size = New System.Drawing.Size(41, 12)
Me.Label1.TabIndex = 4
Me.Label1.Text = "Label1"
'
'Form1
'
Me.AutoScaleDimensions = New System.Drawing.SizeF(6.0!, 12.0!)
Me.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font
Me.ClientSize = New System.Drawing.Size(416, 219)
Me.Controls.Add(Me.GroupBox1)
Me.Name = "Form1"
Me.Text = "Form1"
Me.GroupBox1.ResumeLayout(False)
Me.GroupBox1.PerformLayout()
Me.ResumeLayout(False)
End Sub
Friend WithEvents GroupBox1 As System.Windows.Forms.GroupBox
Friend WithEvents TextBox1 As System.Windows.Forms.TextBox
Friend WithEvents Label1 As System.Windows.Forms.Label
End Class